从键盘上输入若干学生的成绩,计算平均分并输出最高成绩和最低成绩,当输入负数时结束
时间: 2023-11-20 19:54:09 浏览: 99
可以使用while循环语句实现该功能。首先需要定义一个计数器count和一个累加器sum,用于统计输入的成绩个数和总成绩。同时也需要定义一个变量max和min,用于记录最高分和最低分。当输入的成绩小于0时,循环结束,计算平均分并输出最高分和最低分。
代码如下:
```
#include <stdio.h>
int main() {
float score, max, min, sum = 0;
int count = 0;
scanf("%f", &score);
max = min = score;
while (score >= 0) {
count++;
sum += score;
if (score > max) {
max = score;
}
if (score < min) {
min = score;
}
scanf("%f", &score);
}
if (count > 0) {
printf("平均分为:%.2f\n最高成绩为:%.2f\n最低成绩为:%.2f\n", sum / count, max, min);
} else {
printf("未输入成绩\n");
}
return 0;
}
```
相关问题
从键盘输入若干学生的成绩,统计并输出最高成绩、最低成绩和平均成绩,当输入负数时结束输入。
可以使用while循环语句实现从键盘输入若干学生的成绩,统计并输出最高成绩、最低成绩和平均成绩,当输入负数时结束输入。具体步骤如下:
1. 定义变量sum、count、max、min、score,分别表示总成绩、学生数、最高成绩、最低成绩和每个学生的成绩。
2. 使用while循环语句,当输入的成绩大于等于0时,执行以下操作:
a. 输入成绩score。
b. 判断score是否大于max,如果是则将max赋值为score。
c. 判断score是否小于min,如果是则将min赋值为score。
d. 将score加入总成绩sum中。
e. 学生数count加1。
3. 循环结束后,计算平均成绩avg=sum/count。
4. 输出最高成绩max、最低成绩min和平均成绩avg。
代码如下(C语言):
```
#include <stdio.h>
int main() {
float sum = 0, avg, max = 0, min = 100, score;
int count = 0;
printf("请输入学生成绩:\n");
scanf("%f", &score);
while (score >= 0) {
if (score > max) {
max = score;
}
if (score < min) {
min = score;
}
sum += score;
count++;
scanf("%f", &score);
}
if (count > 0) {
avg = sum / count;
printf("最高成绩:%f\n最低成绩:%f\n平均成绩:%f\n", max, min, avg);
} else {
printf("没有输入成绩!\n");
}
return 0;
}
```
从键盘输入若干学生的成绩,统计并输出最高成绩和最低成绩,当输入负数时循环结束
这道题目可以使用while循环语句实现。首先,我们需要从键盘上输入学生成绩n,然后将n的值赋值给max和min。接着,我们需要使用while循环语句,当输入负数时循环结束。在循环中,我们需要将max和min与n做比较,如果n大于max,则将max的值更新为n;如果n小于min,则将min的值更新为n。最后,我们输出最大值和最小值即可。
代码如下所示(C语言):
```
#include <stdio.h>
int main() {
int n, max, min;
printf("输入学生成绩n:");
scanf("%d", &n);
max = n;
min = n;
while (n >= 0) {
if (n > max) max = n;
if (n < min) min = n;
printf("输入学生成绩n:");
scanf("%d", &n);
}
printf("最大为:%d,最小为:%d", max, min);
return 0;
}
```
相关推荐
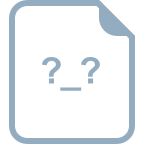












