adaboostpython代码
时间: 2023-08-11 21:07:18 浏览: 77
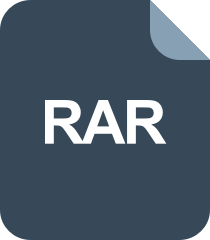
【数据驱动】复杂网络的数据驱动控制附Matlab代码.rar
Adaboost(自适应增强)是一种集成学习方法,用于提高分类算法的准确性。下面是一个使用Python实现Adaboost算法的示例代码:
```python
import numpy as np
class Adaboost:
def __init__(self, n_estimators):
self.n_estimators = n_estimators
self.estimators = []
self.estimator_weights = []
def fit(self, X, y):
# 初始化样本权重
sample_weight = np.ones(X.shape[0]) / X.shape[0]
for _ in range(self.n_estimators):
# 使用权重为样本拟合一个基础分类器
estimator = DecisionTreeClassifier(max_depth=1)
estimator.fit(X, y, sample_weight=sample_weight)
# 计算分类误差率
y_pred = estimator.predict(X)
incorrect = y_pred != y
error = np.sum(sample_weight[incorrect])
# 计算基础分类器的权重
estimator_weight = 0.5 * np.log((1 - error) / error)
# 更新样本权重
sample_weight *= np.exp(estimator_weight * incorrect)
# 归一化样本权重
sample_weight /= np.sum(sample_weight)
# 将基础分类器和权重添加到列表中
self.estimators.append(estimator)
self.estimator_weights.append(estimator_weight)
def predict(self, X):
# 对输入数据进行预测
y_pred = np.zeros(X.shape[0])
for estimator, estimator_weight in zip(self.estimators, self.estimator_weights):
y_pred += estimator_weight * estimator.predict(X)
return np.sign(y_pred)
# 使用示例
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
# 生成一些示例数据
X, y = make_classification(n_samples=100, n_features=10, random_state=42)
# 将数据集划分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 创建Adaboost分类器实例并训练模型
adaboost = Adaboost(n_estimators=10)
adaboost.fit(X_train, y_train)
# 使用训练好的模型进行预测
y_pred = adaboost.predict(X_test)
# 计算准确性
accuracy = accuracy_score(y_test, y_pred)
print("Accuracy:", accuracy)
```
上述代码使用`sklearn`库的`DecisionTreeClassifier`作为基础分类器,并将其用于Adaboost算法。注意,这只是一个简单的示例,真实的Adaboost实现可能会有更多的优化和改进。
阅读全文
相关推荐
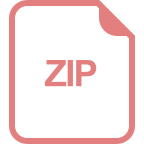
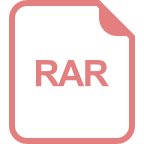
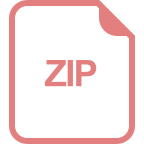
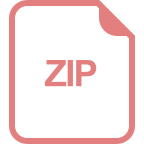
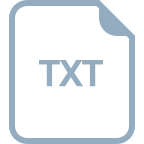
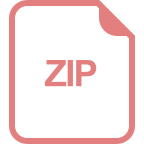
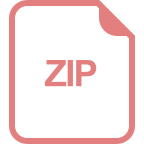
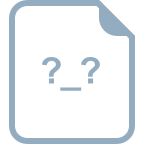
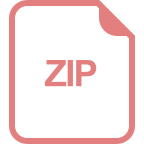
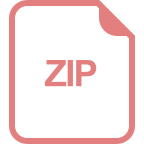
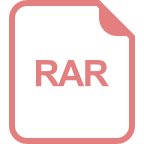
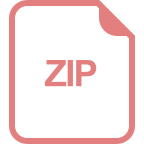