能否用C语言解决该问题
时间: 2024-10-13 21:04:54 浏览: 1
当然可以使用C语言来解决这个问题,不过由于涉及到用户输入处理、条件判断、浮点数运算以及字符串操作等复杂任务,C语言相对其他高级语言可能会显得稍微繁琐一些。以下是C语言的一个简化版实现,它包含了关键步骤,但可能需要更多代码来处理边界检查和错误处理:
```c
#include <stdio.h>
#include <string.h>
#define MAX_NAME_LEN 50
#define MAX_PHONE_LEN 12
#define MIN_BMI 16.0
#define MAX_BMI 40.0
typedef struct {
char name[MAX_NAME_LEN];
char phone[MAX_PHONE_LEN];
int id;
float temp_c;
float temp_f;
float temp_kelvin;
float height_cm;
float weight_kg;
float bmi;
char category[20];
} Patient;
void calculate_and_print(Patient patient) {
// ...(根据上述需求计算各项数值)
}
int main() {
Patient patient;
// 输入部分
fgets(patient.name, sizeof(patient.name), stdin);
fgets(patient.phone, sizeof(patient.phone), stdin);
scanf("%d", &patient.id);
scanf("%f", &patient.temp_c);
// 接下来需要对输入做进一步处理,比如检查长度、范围、非数字字符等
// 计算部分
patient.temp_f = (patient.temp_c * 9.0 / 5.0) + 32;
patient.temp_kelvin = patient.temp_c + 273.15;
patient.height_cm /= 100.0;
patient.bmi = patient.weight_kg / (patient.height_cm * patient.height_cm);
calculate_and_print(patient);
return 0;
}
```
在这个C语言版本中,你需要手动处理用户输入的边界检查和格式化,并且可能需要更多的自定义函数来完成特定的任务,如计算BMI和分类。如果想让代码更易读和维护,可能还需要引入一些辅助函数。
相关推荐
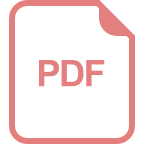














