#4、对于一个形状为(1,28,28,3)的图像数据,尝试使用reshape()、unsqueeze()、squeeze()、transpose()和permute(),将其变为(28,28,3)、(3,28,28)、(28,28)等不同形状请用代码实现
时间: 2024-10-25 22:03:50 浏览: 14
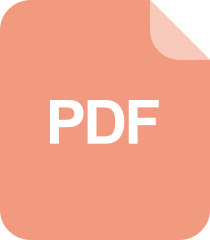
Python reshape的用法及多个二维数组合并为三维数组的实例
首先,让我们理解这些PyTorch中的函数作用:
- `reshape()`: 改变张量的大小,但保持元素总数不变。
- `unsqueeze()`: 在指定维度添加一个尺寸为1的轴。
- `squeeze()`: 删除所有大小为1的维度。
- `transpose()`: 轴之间的元素交换,可以看作是`permute()`的一个特殊情况,通常用于改变矩阵的行和列顺序。
- `permute()`: 完全地重新排列张量的维度。
下面是一个例子,展示了如何使用这些函数将给定形状的图像数据转换成不同的形状:
```python
import torch
# 假设input_data是 (1, 28, 28, 3) 的torch tensor
input_data = torch.randn(1, 28, 28, 3) # 假设它是一个随机生成的数据
# 1. reshape to (28, 28, 3)
reshaped_28x28x3 = input_data.permute(1, 2, 0).contiguous().view(28, 28, 3)
# 2. unsqueeze to add a new dimension at dim=0
unsqueeze_dim0 = input_data.unsqueeze(0) # (1, 28, 28, 3) -> (3, 28, 28, 3)
# 3. squeeze to remove size 1 dimensions
squeezed = input_data.squeeze() # (1, 28, 28, 3) -> (28, 28, 3) if the first dimension is 1
# 4. transpose and permute to change the order of dimensions
transposed = input_data.transpose(1, 2) # (1, 28, 28, 3) -> (1, 28, 3, 28)
permuted = input_data.permute(2, 0, 1) # (1, 28, 28, 3) -> (3, 1, 28, 28)
print(f"Original shape: {input_data.shape}")
print(f"reshaped_28x28x3: {reshaped_28x28x3.shape}")
print(f"unsqueeze_dim0: {unsqueeze_dim0.shape}")
print(f"squeezed: {squeezed.shape} (if squeezed first dim was 1)")
print(f"transposed: {transposed.shape}")
print(f"permuted: {permuted.shape}")
```
请注意,如果输入数据的某些维度原本就是1,直接`squeeze()`可能会丢失信息,除非在之前先确认不需要保留那些维度。同时,`unsqueeze()`和`permute()`/`transpose()`的结果取决于原始数据的具体结构。
阅读全文
相关推荐
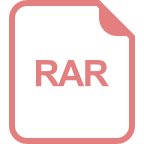
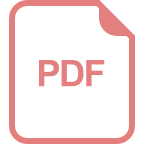
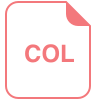
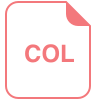
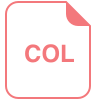



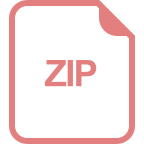
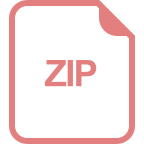
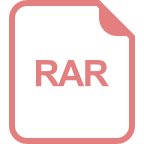