python 实现链表
时间: 2024-01-01 11:22:14 浏览: 33
以下是Python实现单链表的示例代码:
```python
# 定义链表节点类
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
# 定义链表类
class LinkedList:
def __init__(self):
self.head = None
# 在链表末尾添加节点
def addAtTail(self, val: int) -> None:
if not self.head:
self.head = ListNode(val)
else:
cur = self.head
while cur.next:
cur = cur.next
cur.next = ListNode(val)
# 在链表头部添加节点
def addAtHead(self, val: int) -> None:
if not self.head:
self.head = ListNode(val)
else:
new_head = ListNode(val)
new_head.next = self.head
self.head = new_head
# 在指定位置添加节点
def addAtIndex(self, index: int, val: int) -> None:
if index == 0:
self.addAtHead(val)
else:
cur = self.head
for i in range(index - 1):
if not cur:
return
cur = cur.next
if not cur:
return
new_node = ListNode(val)
new_node.next = cur.next
cur.next = new_node
# 删除指定位置的节点
def deleteAtIndex(self, index: int) -> None:
if not self.head:
return
if index == 0:
self.head = self.head.next
else:
cur = self.head
for i in range(index - 1):
if not cur:
return
cur = cur.next
if not cur or not cur.next:
return
cur.next = cur.next.next
# 获取指定位置的节点值
def get(self, index: int) -> int:
if not self.head:
return -1
cur = self.head
for i in range(index):
if not cur:
return -1
cur = cur.next
if not cur:
return -1
return cur.val
# 打印链表
def printList(self) -> None:
if not self.head:
print("Empty List")
else:
cur = self.head
while cur:
print(cur.val, end=" ")
cur = cur.next
print()
# 创建链表并测试
linked_list = LinkedList()
linked_list.addAtHead(1)
linked_list.addAtTail(3)
linked_list.addAtIndex(1, 2)
linked_list.printList() # 输出:1 2 3
linked_list.deleteAtIndex(1)
linked_list.printList() # 输出:1 3
print(linked_list.get(1)) # 输出:3
```
相关推荐
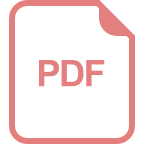














