写一个集群围捕目标的python代码
时间: 2023-04-07 09:03:59 浏览: 109
我可以回答这个问题。以下是一个简单的集群围捕目标的Python代码示例:
```python
import random
# 定义目标位置
target_x = random.randint(0, 100)
target_y = random.randint(0, 100)
# 定义集群中的机器人数量
num_robots = 10
# 定义每个机器人的初始位置和速度
robots = []
for i in range(num_robots):
robot_x = random.randint(0, 100)
robot_y = random.randint(0, 100)
robot_speed = random.randint(1, 5)
robots.append((robot_x, robot_y, robot_speed))
# 定义集群的最大速度
max_speed = 5
# 定义每个机器人的最大转向角度
max_turn_angle = 30
# 定义每个机器人的视野范围
view_range = 20
# 定义每个机器人的视野角度
view_angle = 60
# 定义每个机器人的跟随距离
follow_distance = 10
# 定义每个机器人的跟随角度
follow_angle = 30
# 定义每个机器人的追踪距离
track_distance = 20
# 定义每个机器人的追踪角度
track_angle = 60
# 定义每个机器人的攻击距离
attack_distance = 5
# 定义每个机器人的攻击角度
attack_angle = 10
# 定义每个机器人的状态
# 0 - 搜索目标
# 1 - 跟随目标
# 2 - 追踪目标
# 3 - 攻击目标
robot_states = [0] * num_robots
# 定义每个机器人的目标位置
robot_targets = [(target_x, target_y)] * num_robots
# 定义每个机器人的行动
def robot_action(robot_index):
robot_x, robot_y, robot_speed = robots[robot_index]
target_x, target_y = robot_targets[robot_index]
# 计算机器人到目标的距离和角度
distance = ((target_x - robot_x) ** 2 + (target_y - robot_y) ** 2) ** 0.5
angle = math.atan2(target_y - robot_y, target_x - robot_x) * 180 / math.pi
# 根据机器人的状态进行行动
if robot_states[robot_index] == 0:
# 搜索目标
if distance < view_range and abs(angle) < view_angle / 2:
robot_states[robot_index] = 1
elif robot_states[robot_index] == 1:
# 跟随目标
if distance > follow_distance or abs(angle) > follow_angle / 2:
robot_states[robot_index] = 0
elif distance < track_distance and abs(angle) < track_angle / 2:
robot_states[robot_index] = 2
elif distance < attack_distance and abs(angle) < attack_angle / 2:
robot_states[robot_index] = 3
elif robot_states[robot_index] == 2:
# 追踪目标
if distance > track_distance or abs(angle) > track_angle / 2:
robot_states[robot_index] = 1
elif distance < attack_distance and abs(angle) < attack_angle / 2:
robot_states[robot_index] = 3
elif robot_states[robot_index] == 3:
# 攻击目标
if distance > attack_distance or abs(angle) > attack_angle / 2:
robot_states[robot_index] = 2
# 根据机器人的状态进行移动
if robot_states[robot_index] == 0:
# 搜索目标
robot_turn_angle = random.uniform(-max_turn_angle, max_turn_angle)
robot_speed = random.uniform(0, max_speed)
elif robot_states[robot_index] == 1:
# 跟随目标
robot_turn_angle = angle
robot_speed = min(distance / follow_distance * max_speed, max_speed)
elif robot_states[robot_index] == 2:
# 追踪目标
robot_turn_angle = angle
robot_speed = max_speed
elif robot_states[robot_index] == 3:
# 攻击目标
robot_turn_angle = angle
robot_speed = 0
# 更新机器人的位置和速度
robot_x += robot_speed * math.cos(robot_turn_angle * math.pi / 180)
robot_y += robot_speed * math.sin(robot_turn_angle * math.pi / 180)
robot_speed = min(robot_speed, max_speed)
robot_x = max(0, min(robot_x, 100))
robot_y = max(0, min(robot_y, 100))
robots[robot_index] = (robot_x, robot_y, robot_speed)
# 模拟机器人的运动
for i in range(100):
for j in range(num_robots):
robot_action(j)
```
阅读全文
相关推荐
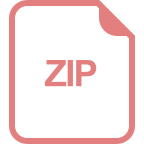
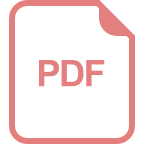
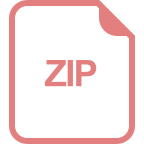
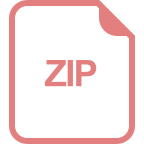
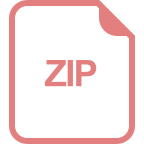
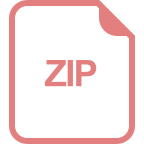
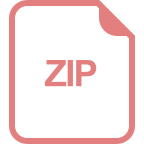
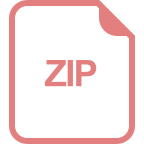
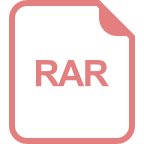
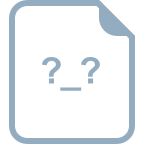
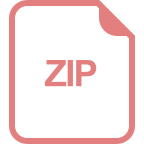
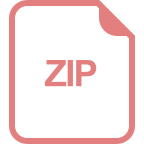
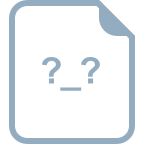
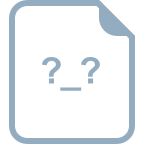
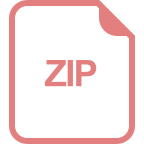
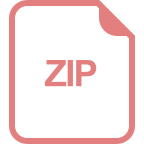