for item in area_dict.items(): ws3.append(item)报错AttributeError: 'list' object has no attribute 'items'
时间: 2023-10-13 15:06:16 浏览: 73
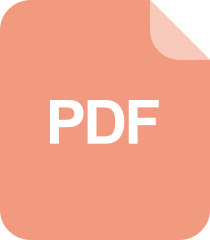
解决运行出现dict object has no attribute has_key问题

这个错误提示意味着你试图在一个列表对象上调用`items()`方法,但是列表对象并没有这个方法。`items()`方法是用于字典对象的。我猜测你的`area_dict`实际上是一个列表,而不是字典。你需要检查一下`area_dict`的类型是否正确。如果`area_dict`确实是一个列表,那么你需要使用`enumerate()`函数来遍历它,而不是`items()`方法。示例代码如下:
```
for idx, item in enumerate(area_dict):
ws3.append((idx, item))
```
这里使用`enumerate()`函数来遍历`area_dict`,同时使用`append()`方法将元组`(idx, item)`添加到`ws3`工作表中。元组中的`idx`表示列表中每个元素的索引,`item`表示列表中的每个元素。
阅读全文
相关推荐
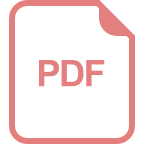
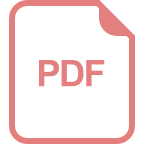















