// This function initializes the PLLCR register. void InitPll(unsigned int val) { volatile unsigned int i; EALLOW; SysCtrlRegs.PLLCR.bit.DIV = val; EDIS; DisableDog(); // Wait PLL lock cycles. for(i= 0; i< ( (131072/2)/12 ); i++) {;} } }
时间: 2024-04-23 11:24:16 浏览: 19
这段代码是用来初始化 PLLCR 寄存器的。PLL(Phase Locked Loop)是一种电路,用于将时钟信号锁定在特定频率上。在这个函数中,首先通过 EALLOW 和 EDIS 宏来使能和禁止写入系统控制寄存器,然后将 PLLCR 寄存器的 DIV 位设置为传入的参数 val。最后,通过一个循环等待 PLL 锁定。这个循环的次数是根据特定的时钟频率计算得出的。
相关问题
x86架构 /boot/grub2/i386-pc/boot.img 文件 源码 并注释
/boot/grub2/i386-pc/boot.img 文件是一个针对x86架构的引导镜像文件,它包含了用于引导启动计算机的程序和参数等信息。
以下是/boot/grub2/i386-pc/boot.img文件的源码注释:
```
/*
* This file contains the source code for the x86 version of the GRUB
* boot loader. It is responsible for loading the operating system kernel
* from the hard disk and transferring control to it.
*/
#include <grub/types.h>
#include <grub/i386/pc/boot.h>
#include <grub/i386/pc/loadfile.h>
/*
* The main function of the boot loader. It is called by the BIOS when the
* computer is powered on or reset. It initializes the hardware, loads the
* kernel from the hard disk, and transfers control to it.
*/
int
main (void)
{
/* Initialize the hardware */
init_hw ();
/* Load the kernel from the hard disk */
if (load_kernel () != 0)
{
/* Error loading the kernel */
return 1;
}
/* Transfer control to the kernel */
transfer_control ();
/* Should never get here */
return 0;
}
/*
* Initializes the hardware, including the console output and disk system.
*/
void
init_hw (void)
{
/* Initialize the console */
init_console ();
/* Initialize the disk system */
init_disk ();
}
/*
* Loads the kernel from the hard disk into memory.
*/
int
load_kernel (void)
{
/* Read the kernel from the hard disk */
if (read_kernel () != 0)
{
/* Error reading the kernel */
return 1;
}
/* Initialize the kernel */
init_kernel ();
return 0;
}
/*
* Transfers control to the kernel by jumping to its entry point.
*/
void
transfer_control (void)
{
/* Jump to the kernel entry point */
jump_to_kernel ();
}
```
以上是x86架构/boot/grub2/i386-pc/boot.img文件的源码和注释。其中,主要包括了引导加载程序的主函数、硬件初始化函数、内核加载函数和转移控制函数等。通过这些函数的调用,boot.img文件可以成功地将计算机引导到操作系统内核。
Initializes the RCC Oscillators according to the specified parameters in the RCC_OscInitTypeDef structure.是什么意思
"Initializes the RCC Oscillators according to the specified parameters in the RCC_OscInitTypeDef structure." 意思是根据 RCC_OscInitTypeDef 结构体中指定的参数初始化 RCC 振荡器。
在 STM32 系列芯片中,RCC(Reset and Clock Control)模块负责控制芯片的复位和时钟。其中,时钟的产生需要依赖于外部晶振或内部 RC 振荡器等振荡器,因此在使用时需要先对这些振荡器进行初始化。
RCC_OscInitTypeDef 结构体中包含了对振荡器进行初始化的参数,通过调用相应的函数并传递 RCC_OscInitTypeDef 结构体作为参数,就可以对芯片的振荡器进行初始化。这句话的意思就是根据 RCC_OscInitTypeDef 中的参数对 RCC 振荡器进行初始化。
相关推荐
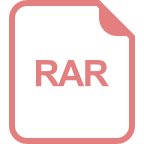
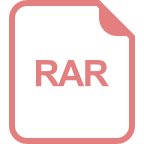
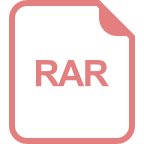













