opencv图像中余弦噪声去除,使用c++代码
时间: 2023-11-14 20:15:02 浏览: 53
以下是一个简单的C++代码,用于去除OpenCV图像中的余弦噪声:
```
#include <opencv2/opencv.hpp>
#include <iostream>
#include <cmath>
using namespace cv;
using namespace std;
int main()
{
Mat image = imread("image.jpg", IMREAD_GRAYSCALE);
if (image.empty())
{
cout << "Could not open or find the image" << endl;
return -1;
}
int rows = image.rows;
int cols = image.cols;
// 构建余弦波图像
Mat cosImage(rows, cols, CV_32FC1);
float frequency = 10.0f;
for (int i = 0; i < rows; i++)
{
float* p = cosImage.ptr<float>(i);
for (int j = 0; j < cols; j++)
{
p[j] = cos(2 * CV_PI * frequency * j / cols);
}
}
// 对图像进行傅里叶变换
Mat dctImage;
dct(image, dctImage, DCT_ROWS);
// 对余弦波进行傅里叶变换
Mat dctCosImage;
dct(cosImage, dctCosImage, DCT_ROWS);
// 除去余弦噪声
Mat filteredDctImage(rows, cols, CV_32FC1);
for (int i = 0; i < rows; i++)
{
float* p = dctImage.ptr<float>(i);
float* q = dctCosImage.ptr<float>(i);
float* r = filteredDctImage.ptr<float>(i);
for (int j = 0; j < cols; j++)
{
r[j] = p[j] / q[j];
}
}
// 对处理后的图像进行傅里叶逆变换
Mat filteredImage;
idct(filteredDctImage, filteredImage, DCT_ROWS);
// 将图像显示出来
imshow("Original Image", image);
imshow("Filtered Image", filteredImage);
waitKey();
return 0;
}
```
这段代码首先读取了一个灰度图像,然后构建了一个余弦波图像。接着,它对原始图像和余弦波图像分别进行了傅里叶变换,并将它们相除以消除余弦噪声。最后,它对处理后的图像进行了傅里叶逆变换,并将其显示出来。
相关推荐
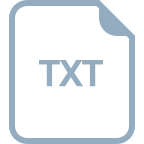
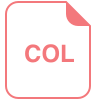
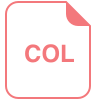
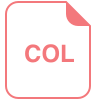
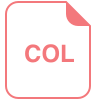
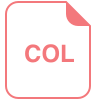







