c语言用一个队列实现栈
时间: 2023-10-14 17:04:50 浏览: 122
可以用一个队列来实现栈的操作。实现方式如下:
1. 定义一个队列,将其当做栈来使用。
2. 入栈操作:将元素插入队列的队尾。
3. 出栈操作:将队列中前面的元素依次出队并插入队列的队尾,直到队列中只剩下一个元素,这个元素即为栈顶元素,将其出队即可。
4. 获取栈顶元素:同出栈操作,只需不将栈顶元素出队即可。
下面是代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
typedef struct {
int data[MAX_SIZE];
int front;
int rear;
} Queue;
void init(Queue *q) {
q->front = q->rear = 0;
}
int is_empty(Queue *q) {
return q->front == q->rear;
}
int is_full(Queue *q) {
return (q->rear + 1) % MAX_SIZE == q->front;
}
void push(Queue *q, int x) {
if (is_full(q)) {
printf("stack overflow!\n");
return;
}
q->data[q->rear] = x;
q->rear = (q->rear + 1) % MAX_SIZE;
}
int pop(Queue *q) {
if (is_empty(q)) {
printf("stack underflow!\n");
exit(1);
}
int x = q->data[q->front];
q->front = (q->front + 1) % MAX_SIZE;
return x;
}
int top(Queue *q) {
if (is_empty(q)) {
printf("stack underflow!\n");
exit(1);
}
return q->data[q->front];
}
int main() {
Queue q;
init(&q);
push(&q, 1);
push(&q, 2);
push(&q, 3);
printf("%d\n", top(&q)); // 输出 3
printf("%d\n", pop(&q)); // 输出 3
printf("%d\n", top(&q)); // 输出 2
push(&q, 4);
printf("%d\n", top(&q)); // 输出 4
return 0;
}
```
阅读全文
相关推荐
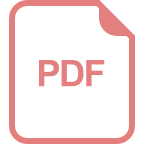
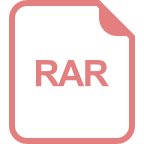
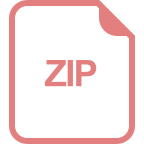
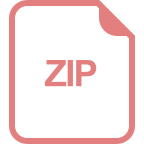
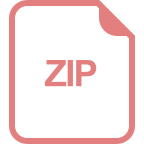
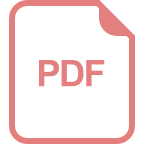
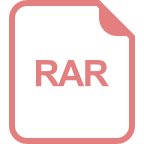
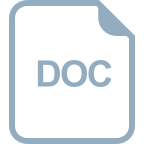
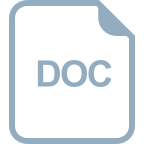
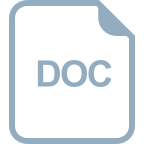
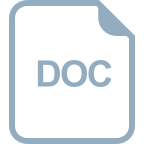
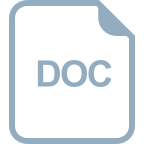