分析 friend istream& operator>>(istream&, Fraction& f)
时间: 2024-08-15 08:02:43 浏览: 46
`friend istream& operator>>(istream&, Fraction& f)` 这行代码是一个友元函数声明,它允许 `istream` 类型的对象直接访问 `Fraction` 类的实例变量,并参与到输入流操作中。这里的 `Fraction` 是一个类,代表分数,而 `operator>>` 是用于从输入流(如标准输入 `cin`)读取数据到这个 `Fraction` 对象的赋值运算符重载。
简而言之,当你在程序中使用 `cin >> f` 的时候,实际上是通过这个友元函数将用户的输入转换并存储到 `Fraction` 对象 `f` 中。这是一种方便的编程技巧,可以使输入流操作对类的内部细节透明,提高了代码的可读性和易用性。
相关问题
class Fraction { public: Fraction(); //缺省构造函数设置分子为0,分母为1 Fraction(int n); //只有1 个参数,设置分子为n,分母为1 Fraction(int n, int d); //设置分子为n,分母为d void setValue(int n, int d); //设置分子和分母 int getNum(); //获取分子值 int getDen(); //获取分母值 double getDoubleValue(); //获取分数对应的小数值 Fraction add(const Fraction & f2); //分数相加 Fraction operator*(const Fraction &f2); //分数相加 void output(); //按分数形式显示最简分数,按真分数或带分数形式输出,不要有多余的空格 friend ostream &operator<<(ostream &out, Fraction &f);//重载输出,分数形式显示最简分数,按真分数或带分数形式输出,不要有多余的空格 friend istream &operator>>(istream &in, Fraction &f); //输入重载 private: int num; //分子 int den; //分母 void normalize();//规范化 int gcd(); //求最大公约数 };
这是一个分数类的定义,其中包含了三种构造函数:缺省构造函数、只有一个参数的构造函数和两个参数的构造函数。还有一些成员函数,如设置分子和分母的函数、获取分子和分母的函数、获取分数对应的小数值的函数、分数相加的函数、重载输出的函数和输入重载的函数等。其中,分数相加的函数和重载输出的函数都使用了运算符重载来实现。此外,还有一些私有函数,如规范化函数和求最大公约数的函数。
write an overloaded friend ostream insertion operator function that allows a Fraction object to be output and appear in the form num/denom, using a cout statement. b. Write an overloaded friend istream extraction operator function that allows fractions in the form a/b to be input and assigned to a Fraction object.
Sure, I can help you with that!
Here is the implementation of the overloaded friend ostream insertion operator function for the Fraction class:
```c++
#include <iostream>
class Fraction {
private:
int numerator;
int denominator;
public:
Fraction(int num, int denom) : numerator(num), denominator(denom) { }
friend std::ostream& operator<<(std::ostream& os, const Fraction& frac);
};
std::ostream& operator<<(std::ostream& os, const Fraction& frac) {
os << frac.numerator << "/" << frac.denominator;
return os;
}
```
And here is the implementation of the overloaded friend istream extraction operator function for the Fraction class:
```c++
#include <iostream>
#include <sstream>
class Fraction {
private:
int numerator;
int denominator;
public:
Fraction(int num, int denom) : numerator(num), denominator(denom) { }
friend std::istream& operator>>(std::istream& is, Fraction& frac);
};
std::istream& operator>>(std::istream& is, Fraction& frac) {
std::string input;
is >> input;
std::stringstream ss(input);
int num, denom;
char slash;
ss >> num >> slash >> denom;
frac.numerator = num;
frac.denominator = denom;
return is;
}
```
You can use these overloaded operators to output and input Fraction objects as follows:
```c++
int main() {
Fraction f1(3, 4);
std::cout << f1 << std::endl; // outputs "3/4"
Fraction f2(0, 1);
std::cin >> f2; // inputs a Fraction from standard input in the form "num/denom"
std::cout << f2 << std::endl; // outputs the input Fraction
return 0;
}
```
I hope this helps! Let me know if you have any other questions.
阅读全文
相关推荐
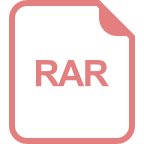
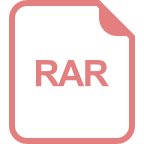
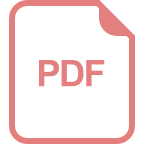
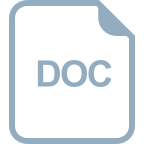




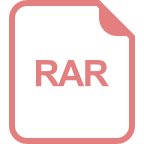
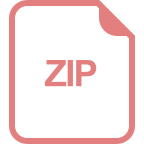
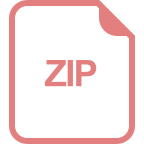
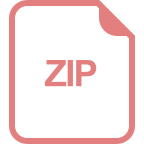
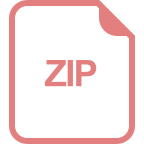
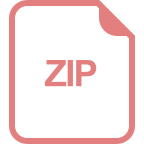