如何使用beautifulsoup爬取表格中“项目名称"的数据,如果表格中找到则写入字典中,如果表格没"项目名称"则向字典写入空值
时间: 2024-10-21 10:10:56 浏览: 34
在Python中,你可以使用BeautifulSoup库配合requests库来抓取网页中的数据,特别是HTML表格中的内容。以下是一个简单的步骤指南:
1. **安装依赖**:
首先,你需要确保已经安装了`requests`和`beautifulsoup4`库。如果没有,可以使用`pip`安装:
```
pip install requests beautifulsoup4
```
2. **发送请求获取HTML**:
使用`requests.get()`函数获取包含表格的网页源码:
```python
import requests
url = 'http://example.com' # 替换为你需要爬取的网页URL
response = requests.get(url)
if response.status_code == 200: # 检查请求是否成功
html_content = response.text
else:
print("请求失败")
return
```
3. **解析HTML**:
使用BeautifulSoup解析HTML内容:
```python
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
table = soup.find('table') # 找到目标表格
if table is not None: # 如果表格存在
rows = table.find_all('tr') # 获取所有行
data_dict = {}
for row in rows:
cols = row.find_all('td') or row.find_all('th') # td用于内容,th用于标题
if len(cols) > 0 and "项目名称" in cols[0].text.lower(): # 检查第一列是否是项目名称
project_name = cols[0].get_text().strip() # 提取项目名称
data_dict[row] = {"项目名称": project_name} # 将数据写入字典
else:
data_dict[row] = {} # 如果无项目名称,则添加空值
else:
data_dict = {} # 表格不存在,直接创建空字典
print(data_dict)
```
4. **异常处理**:
可能会遇到网络错误、解析错误等,记得加上适当的错误处理。
如果你发现某个网站有反爬虫机制,可能需要考虑设置延迟、模拟用户代理或使用更复杂的方法(如Selenium)。注意遵守网站的robots.txt规则并尊重版权。
阅读全文
相关推荐
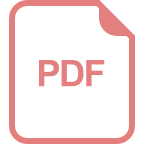
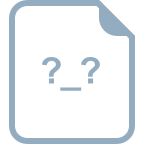
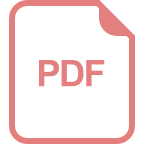
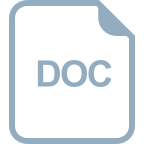
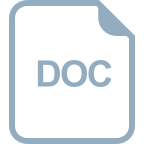













