用python根据文档内容,统计出频度最高的二十个关键词,并绘出关键词云图
时间: 2024-12-26 18:31:18 浏览: 12
在Python中,我们可以使用`nltk`库(Natural Language Toolkit)来进行文本处理和关键词频率统计,然后结合`wordcloud`库绘制关键词云图。以下是一个基本步骤:
1. **安装所需库**:
```bash
pip install nltk wordcloud matplotlib
```
2. **导入模块**:
```python
import nltk
from nltk.corpus import stopwords
from collections import Counter
from wordcloud import WordCloud, STOPWORDS
import matplotlib.pyplot as plt
```
3. **加载文本数据** (假设是从文件读取):
```python
with open('your_document.txt', 'r') as file:
text = file.read().lower()
```
4. **预处理文本**:
- 分词(Tokenization)
- 移除停用词(如“的”、“是”等常见词汇)
- 词干提取或lemmatization(如果需要的话)
```python
nltk.download('punkt')
nltk.download('stopwords')
stop_words = set(stopwords.words('chinese')) # 对于中文文本
words = [word for word in nltk.word_tokenize(text) if word.isalnum() and not word in stop_words]
```
5. **统计关键词频率**:
```python
counter = Counter(words)
top_keywords = counter.most_common(20)
```
6. **绘制关键词云图**:
```python
def generate_wordcloud(data, title=None):
wordcloud = WordCloud(font_path='simhei.ttf', width=800, height=400,
background_color='white').generate_from_frequencies(data)
plt.figure(figsize=(10, 5))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
if title:
plt.title(title)
plt.show()
generate_wordcloud(dict(top_keywords), "Top 20 Keywords")
```
在这个过程中,你需要替换`'your_document.txt'`为你实际的文档路径,同时确保字体文件(`simhei.ttf`)位于Python脚本目录下,因为WordCloud可能需要特定字体才能显示汉字。
阅读全文
相关推荐
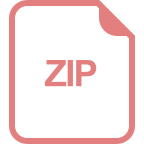
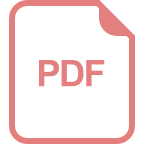
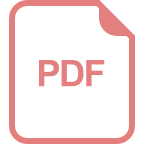















