怎样用spfile启动数据库
时间: 2023-11-18 22:05:58 浏览: 35
要求:使用Flink语言实现一个热门页面统计程序,统计最近1小时内访问量最大的10个页面。
解决方案:
1. 数据源选择:从Kafka中读取网站访问日志数据。
2. 数据清洗:对读取的数据进行清洗,过滤掉无效数据,如请求资源不存在、请求参数错误等。
3. 数据转换:将清洗后的数据转换为页面访问次数的形式,即将每个页面的访问次数累加起来。
4. 窗口统计:使用滑动窗口对数据进行统计,窗口大小为1小时,滑动步长为5分钟。在每个窗口内,计算每个页面的访问量,并按照访问量从大到小排序。
5. TopN筛选:对每个窗口内的数据按照访问量排序后,只保留前10个页面。
6. 数据输出:将统计结果输出到Kafka中,供后续处理使用。
代码实现:
```java
public class HotPageJob {
public static void main(String[] args) throws Exception {
// 设置执行环境
StreamExecutionEnvironment env = StreamExecutionEnvironment.getExecutionEnvironment();
env.setStreamTimeCharacteristic(TimeCharacteristic.EventTime);
env.getConfig().setAutoWatermarkInterval(1000L);
// 读取Kafka数据源
Properties props = new Properties();
props.setProperty("bootstrap.servers", "localhost:9092");
props.setProperty("group.id", "hot-page-group");
FlinkKafkaConsumer<String> kafkaSource = new FlinkKafkaConsumer<>("page-views", new SimpleStringSchema(), props);
kafkaSource.assignTimestampsAndWatermarks(new PageViewTimestampExtractor());
// 数据清洗
DataStream<PageView> pageViews = env.addSource(kafkaSource)
.map(new PageViewParser())
.filter(pageView -> pageView != null);
// 数据转换
DataStream<Tuple2<String, Long>> pageViewCounts = pageViews
.keyBy(PageView::getPage)
.timeWindow(Time.hours(1), Time.minutes(5))
.aggregate(new PageViewCounter(), new PageViewAggregator())
.keyBy(0)
.process(new TopNFilter(10));
// 数据输出
FlinkKafkaProducer<String> kafkaSink = new FlinkKafkaProducer<>("hot-pages", new SimpleStringSchema(), props);
pageViewCounts.map(new PageViewFormatter()).addSink(kafkaSink);
// 执行任务
env.execute("Hot Page Job");
}
// 自定义时间戳提取器,从日志数据中解析出事件时间
public static class PageViewTimestampExtractor extends BoundedOutOfOrdernessTimestampExtractor<String> {
public PageViewTimestampExtractor() {
super(Time.seconds(10));
}
@Override
public long extractTimestamp(String logEntry) {
return PageViewParser.parseTimestamp(logEntry);
}
}
// 自定义解析器,从日志数据中解析出页面访问信息
public static class PageViewParser implements MapFunction<String, PageView> {
private static final Pattern LOG_ENTRY_PATTERN = Pattern.compile("(\\S+)\\s+(\\S+)\\s+\\[(.+?)\\]\\s+\"(\\S+)\\s+(\\S+)\\s+(\\S+)\"\\s+(\\S+)\\s+(\\S+)\\s+\"(.*?)\"\\s+\"(.*?)\"");
@Override
public PageView map(String logEntry) throws Exception {
Matcher matcher = LOG_ENTRY_PATTERN.matcher(logEntry);
if (matcher.matches()) {
String page = matcher.group(6);
long timestamp = parseTimestamp(logEntry);
return new PageView(page, timestamp);
}
return null;
}
public static long parseTimestamp(String logEntry) {
Matcher matcher = LOG_ENTRY_PATTERN.matcher(logEntry);
if (matcher.matches()) {
String timestampStr = matcher.group(3);
SimpleDateFormat format = new SimpleDateFormat("dd/MMM/yyyy:HH:mm:ss Z", Locale.ENGLISH);
try {
Date date = format.parse(timestampStr);
return date.getTime();
} catch (ParseException e) {
e.printStackTrace();
}
}
return -1;
}
}
// 自定义累加器,将每个页面的访问次数累加起来
public static class PageViewCounter implements AggregateFunction<PageView, Long, Long> {
@Override
public Long createAccumulator() {
return 0L;
}
@Override
public Long add(PageView pageView, Long count) {
return count + 1;
}
@Override
public Long getResult(Long count) {
return count;
}
@Override
public Long merge(Long a, Long b) {
return a + b;
}
}
// 自定义聚合器,将每个页面的访问次数累加起来
public static class PageViewAggregator implements WindowFunction<Long, Tuple2<String, Long>, String, TimeWindow> {
@Override
public void apply(String page, TimeWindow window, Iterable<Long> counts, Collector<Tuple2<String, Long>> out) throws Exception {
long count = counts.iterator().next();
out.collect(new Tuple2<>(page, count));
}
}
// 自定义过滤器,对每个窗口内的数据按照访问量排序后,只保留前N个页面
public static class TopNFilter extends KeyedProcessFunction<Tuple, Tuple2<String, Long>, Tuple2<String, Long>> {
private final int n;
private final PriorityQueue<Tuple2<String, Long>> queue;
public TopNFilter(int n) {
this.n = n;
this.queue = new PriorityQueue<>(n, Comparator.comparingLong(Tuple2::f1));
}
@Override
public void processElement(Tuple2<String, Long> pageViewCount, Context context, Collector<Tuple2<String, Long>> out) throws Exception {
queue.offer(pageViewCount);
if (queue.size() > n) {
queue.poll();
}
}
@Override
public void onTimer(long timestamp, OnTimerContext context, Collector<Tuple2<String, Long>> out) throws Exception {
while (!queue.isEmpty()) {
out.collect(queue.poll());
}
}
@Override
public void open(Configuration parameters) throws Exception {
super.open(parameters);
// 注册定时器,每隔5分钟触发一次
long interval = 5 * 60 * 1000L;
long now = System.currentTimeMillis();
long next = now - (now % interval) + interval;
context().timerService().registerProcessingTimeTimer(next);
}
}
// 自定义格式化器,将统计结果转换为字符串形式
public static class PageViewFormatter implements MapFunction<Tuple2<String, Long>, String> {
@Override
public String map(Tuple2<String, Long> pageViewCount) throws Exception {
return String.format("%s,%d", pageViewCount.f0, pageViewCount.f1);
}
}
// 页面访问信息类
public static class PageView {
private final String page;
private final long timestamp;
public PageView(String page, long timestamp) {
this.page = page;
this.timestamp = timestamp;
}
public String getPage() {
return page;
}
public long getTimestamp() {
return timestamp;
}
}
}
```
相关推荐
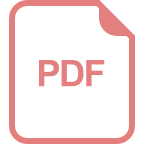
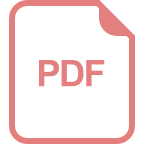














