C#二维向量叉乘代码
时间: 2023-11-30 15:43:10 浏览: 47
根据引用[2]中的公式,C#中二维向量的叉积可以通过以下代码实现:
```csharp
public static float Cross(Vector2 a, Vector2 b)
{
return a.x * b.y - a.y * b.x;
}
```
其中,`Vector2`是Unity中的二维向量类型,`a`和`b`分别表示两个二维向量。函数返回值为两个向量的叉积结果。
相关问题
java二维向量叉乘求交点代码
以下是 Java 中二维向量叉乘求交点的示例代码:
```java
public class Vector2D {
public double x, y;
public Vector2D(double x, double y) {
this.x = x;
this.y = y;
}
// 向量叉乘
public static double crossProduct(Vector2D a, Vector2D b) {
return a.x * b.y - a.y * b.x;
}
// 求交点
public static Vector2D getIntersectionPoint(Vector2D p1, Vector2D v1, Vector2D p2, Vector2D v2) {
double t = crossProduct(new Vector2D(p2.x - p1.x, p2.y - p1.y), v2) / crossProduct(v1, v2);
double x = p1.x + v1.x * t;
double y = p1.y + v1.y * t;
return new Vector2D(x, y);
}
}
```
其中,`Vector2D` 类表示二维向量,包含两个属性 `x`、`y` 和两个方法 `crossProduct`、`getIntersectionPoint`,分别对应向量叉乘、求交点。使用时,可以先实例化两个向量 `p1` 和 `v1`,以及另外两个向量 `p2` 和 `v2`,然后调用 `getIntersectionPoint` 方法得到它们的交点。
二维向量叉乘运算公式
二维向量的叉乘运算公式可以通过三维向量的叉乘公式来推导得出。对于二维向量a和b,我们可以将它们看作三维向量的一个特殊情况,其中z轴的分量为0。即a = [a1, a2, 0]和b = [b1, b2, 0]。那么它们的叉乘a × b就可以表示为:
a × b = [a1, a2, 0] × [b1, b2, 0]
根据三维向量的叉乘公式,我们可以将它展开为:
a × b = [0, 0, a1*b2 - a2*b1]
由于z轴分量为0,所以上述结果中的前两个分量为0。因此,二维向量的叉乘运算公式为:
a × b = a1*b2 - a2*b1
这就是二维向量叉乘的运算公式。
相关推荐
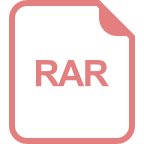
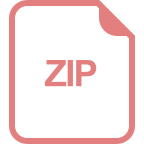
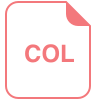











