#include<stdio.h> #include<string.h> struct student { int id; int age; }; int main() { struct student stu1; struct student *p1 = &stu1; scanf("%d %d",p1->age,p1->id); printf("%d %d",p1->age,p1->id); return 0; } 这串代码哪里错了
时间: 2024-01-13 21:05:22 浏览: 39
这段代码有两处错误:
1. scanf() 函数中应该传入参数的地址,而不是参数的值。因此,应该将第 10 行的 scanf() 函数修改为:
```c
scanf("%d %d", &p1->age, &p1->id);
```
2. 在第 11 行中,应该使用箭头运算符 -> 访问结构体指针中的成员,而不是使用点运算符 .。因此,应该将第 11 行的 printf() 函数修改为:
```c
printf("%d %d", p1->age, p1->id);
```
修改后的代码如下:
```c
#include<stdio.h>
#include<string.h>
struct student {
int id;
int age;
};
int main() {
struct student stu1;
struct student *p1 = &stu1;
scanf("%d %d", &p1->age, &p1->id);
printf("%d %d", p1->age, p1->id);
return 0;
}
```
相关问题
#include<stdio.h> #include<string.h> struct student{ char name[100]; int age; }; int main(void) { int i,j; struct student arr[5]; for(i=0;i<5;i++) { scanf("%s%d",arr[i].name,&arr[i].age); } for(i=0;i<4;i++) {
在代码中,最后一个for循环没有结束,缺少右括号。下面是修改后的代码:
```
#include<stdio.h>
#include<string.h>
struct student{
char name[100];
int age;
};
int main(void) {
int i,j;
struct student arr[5];
for(i=0;i<5;i++) {
scanf("%s%d",arr[i].name,&arr[i].age);
}
for(i=0;i<4;i++) {
for(j=0;j<4-i;j++) {
if(arr[j].age>arr[j+1].age) {
struct student t=arr[j];
arr[j]=arr[j+1];
arr[j+1]=t;
}
}
}
for(i=0;i<5;i++) {
printf("%s %d\n",arr[i].name,arr[i].age);
}
return 0; //需要加上返回值
} //需要加上右括号
修改程序:#include <stdio.h> #include <stdlib.h> #include <string.h> #define Element 6 struct Student { char name[8]; char id[12]; int sex; int age; float total_gpa; } student[Element]; int main() { int i; FILE *fp; if ((fp = fopen("stu.dat", "rb")) == NULL) { printf("Can't open file!\n"); exit(0); } for(i=0;i<Element;i++) { fread(&student[i],sizeof(struct student_type),6,fp); printf("%-10s% %4s %4d %4d %-15f\n",student[i].id, student[i].name, student[i].sex, student[i].age, student[i].total_gpa) } fclose(fp); return 0; }
修改程序如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define Element 6
struct Student {
char name[8];
char id[12];
int sex;
int age;
float total_gpa;
} student[Element];
int main() {
int i;
FILE *fp;
if ((fp = fopen("stu.dat", "rb")) == NULL) {
printf("Can't open file!\n");
exit(0);
}
for(i = 0; i < Element; i++) {
fread(&student[i], sizeof(struct Student), 1, fp);
printf("%-10s %-8s %4d %4d %-15f\n", student[i].id, student[i].name, student[i].sex, student[i].age, student[i].total_gpa);
}
fclose(fp);
return 0;
}
```
修改点如下:
1. 结构体名称应该与定义的一致,即 `student` 而不是 `student_type`;
2. 在 `fread` 中,`sizeof(struct student_type)` 应该改为 `sizeof(struct Student)`,且第三个参数应该为 1 而不是 6;
3. 在 `printf` 中,`% %4s` 应该改为 `%-8s`,即输出 `name` 时保证对齐;
4. `printf` 格式化字符串中,`%-10s` 后应该有一个空格,即输出 `id` 时保证对齐。
相关推荐
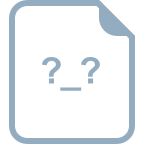
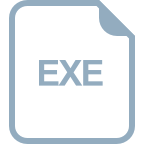
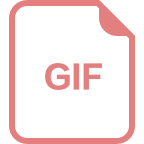













