#define _CRT_SECURE_NO_WARNINGS #include<stdio.h> #include<stdlib.h> #include <string.h> typedef struct student { char num[50]; char name[20]; char sex[4]; char age[4]; }Student; int main() { Student stu = {"202230000630\n","yxy\n" ,"F\n","19\n"}; FILE* fp; if ((fp = fopen("student.dat", "w+")) == NULL) { printf("can not open the file. "); } else { fputs(stu.num, fp); fputs(stu.name, fp); fputs(stu.sex, fp); fputs(stu.age, fp); } fclose(fp); fp = fopen(fp, "r"); if (fp != NULL) { printf("can not open the file. "); }else{ fgets(fp,500,fp); fclose(fp); printf("%s\n", stu.num); printf("%s\n", stu.name); printf("%s\n", stu.sex); printf("%s\n", stu.age);//屏幕显示串信息 } system("pause"); }
时间: 2024-01-23 18:04:17 浏览: 156
这段代码存在一些问题,可以尝试进行以下改进:
1. 在定义结构体时,将年龄的数据类型改为整型,而不是字符型,更符合实际情况。
2. 在文件读取的时候,应该先判断文件是否成功打开,而不是直接使用 `fp` 作为文件名进行打开。
3. 在文件读取的时候,应该使用一个新的变量来存储读取的字符串,而不是直接使用 `fp`,因为 `fp` 是一个 `FILE*` 类型的指针,无法存储字符串。
4. 在文件读取的时候,应该将读取的字符串打印出来,而不是直接打印结构体中的信息,因为结构体中的信息并没有被修改。
改进后的代码如下:
```
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct student {
char num[50];
char name[20];
char sex[4];
int age;
} Student;
int main() {
Student stu = {"202230000630\n", "yxy\n", "F\n", 19};
FILE* fp;
if ((fp = fopen("student.dat", "w+")) == NULL) {
printf("can not open the file.");
} else {
fputs(stu.num, fp);
fputs(stu.name, fp);
fputs(stu.sex, fp);
fprintf(fp, "%d\n", stu.age);
}
fclose(fp);
if ((fp = fopen("student.dat", "r")) == NULL) {
printf("can not open the file.");
} else {
char buffer[500];
fgets(buffer, 500, fp);
strcpy(stu.num, buffer);
fgets(buffer, 500, fp);
strcpy(stu.name, buffer);
fgets(buffer, 500, fp);
strcpy(stu.sex, buffer);
fscanf(fp, "%d", &stu.age);
fclose(fp);
printf("%s", stu.num);
printf("%s", stu.name);
printf("%s", stu.sex);
printf("%d\n", stu.age);
}
system("pause");
}
```
阅读全文
相关推荐
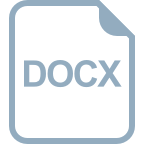
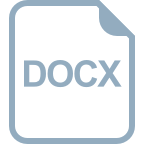
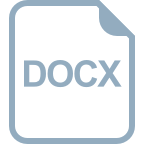















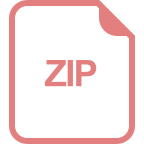
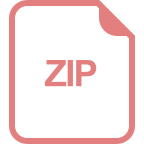