stm32 printf重定向函数怎么写
时间: 2023-06-22 07:21:34 浏览: 177
在使用STM32的printf输出时,需要重定向printf函数,将其输出到UART等外设上。下面是一个简单的重定向函数的例子:
```c
#include <stdio.h>
#include "stm32f10x.h" // 根据具体芯片型号更改头文件
int fputc(int ch, FILE *f) {
USART_SendData(USART1, (uint8_t) ch); // 将字符发送到USART1
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET); // 等待发送完成
return ch;
}
```
在这个例子中,我们使用了STM32的USART1模块作为输出设备,将所有字符都发送到USART1中。我们可以将这个函数保存到一个名为“printf-redirect.c”的文件中,然后在需要使用printf的地方包含这个文件即可。例如,在main函数中:
```c
#include "stdio-redirect.h"
int main() {
// 初始化USART1
// ...
// 重定向printf
printf("Hello, world!");
while(1);
}
```
在这个例子中,我们在main函数中调用了printf函数,由于我们已经在“printf-redirect.c”文件中定义了重定向函数fputc,所以所有的输出都会被重定向到USART1模块上。
相关问题
stm32printf重定向
在STM32中,可以使用重定向printf函数来将printf函数的输出重定向到其他设备,比如串口或者LCD显示屏。下面是一个基本的重定向printf函数的例子:
```c
#include <stdio.h>
#include "stm32f10x.h"
int fputc(int ch, FILE *f) {
USART_SendData(USART1, (uint8_t) ch);
while(USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
return ch;
}
```
这个函数将每个字符都发送到USART1串口,并等待发送完成后返回发送的字符。要使用这个重定向函数,需要在使用printf函数之前调用以下代码:
```c
USART_InitTypeDef USART_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
// Enable GPIOA clock
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
// Enable USART1 clock
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
// Configure USART1 Tx pin as alternate function push-pull
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// Configure USART1 Rx pin as input floating
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// Configure USART1
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
// Enable USART1
USART_Cmd(USART1, ENABLE);
// Redirect printf output to USART1
printf("Hello, world!");
```
这段代码首先配置了USART1串口,然后调用了printf函数,将输出重定向到了USART1串口。最后,在使用完printf函数后,需要记得将printf函数的输出重定向恢复到标准输出。
stm32 printf重定向
stm32是一种微控制器,可以用来设计嵌入式系统。在stm32的开发过程中,printf函数是一个必不可少的工具。然而,stm32没有内置的printf函数,需要使用重定向技术来将printf输出到串口等外设上。
stm32的printf重定向是指将printf函数的输出重定向到另一个设备上,例如串口。为了实现printf重定向,需要编写一个重定向函数。在这个函数中,可以将printf的输出通过串口发送出去。重定向函数的编写需要在项目的初始化函数中完成。
重定向函数的代码如下:
```
#include "stdio.h"
int fputc(int ch, FILE *f)
{
while(!USART_GetFlagStatus(USART1, USART_FLAG_TXE));
USART_SendData(USART1, (uint8_t) ch);
return ch;
}
```
这个函数将在stm32中替代原有的fputc函数。在这个函数中,使用了stm32的串口发送函数USART_SendData(),将printf的输出发送到串口上。同时,为了实现阻塞式输出,使用了循环等待串口发送空闲的语句,避免丢失数据。
需要注意的是,为了使用这个重定向函数,还需要在引入stdio.h头文件,并在main函数中添加如下代码:
```
#include "stdio.h"
int main(void)
{
// 初始化代码
printf("Hello World");
}
```
总之,利用stm32的printf重定向技术,可以将printf输出到不同的设备上,为嵌入式系统设计提供了更多的灵活性和选择。
阅读全文
相关推荐
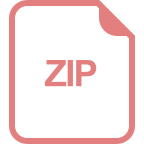


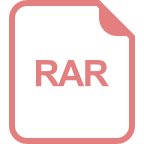
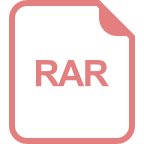
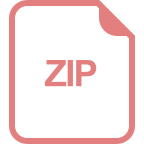
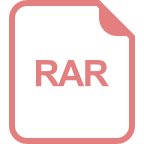
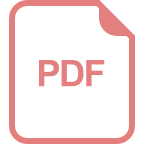
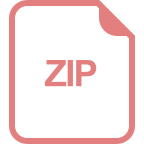
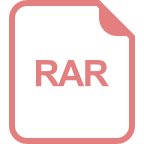




