设计一个python程序,会在白色屏幕中央生成一个半径为200的圆,同时在圆上有直角扇形标识为G1,并且在圆中随机生成一个黑点。按下F键后,程序会计算黑点距离圆心的距离和圆心角度,并将G1扇形移动到黑点处,颜色变为红色。同时,程序会在控制台输出黑点距离圆心距离和圆心角度
时间: 2024-06-03 17:08:29 浏览: 110
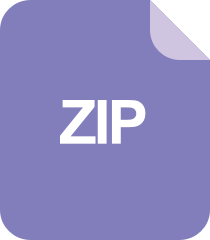
基于Python的校园学生一卡通管理系统.zip

以下是实现该程序的代码:
```python
import pygame
import math
import random
# 初始化pygame
pygame.init()
# 设置屏幕大小和标题
screen = pygame.display.set_mode((600, 600))
pygame.display.set_caption("Move G1 to Black Dot")
# 定义颜色
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
# 声明字体对象
font = pygame.font.Font(None, 28)
# 创建圆和扇形的surface对象
circle_surf = pygame.Surface((400, 400))
circle_surf.set_colorkey(BLACK)
circle_surf.fill(BLACK)
pygame.draw.circle(circle_surf, WHITE, (200, 200), 200)
g1_surf = pygame.Surface((400, 400))
g1_surf.set_colorkey(BLACK)
g1_surf.fill(BLACK)
pygame.draw.arc(g1_surf, RED, (0, 0, 400, 400), math.pi/2, math.pi, 0)
pygame.draw.line(g1_surf, RED, (200, 200), (200, 0), 5)
# 随机生成黑点
dot_x = random.randint(0, 400)
dot_y = random.randint(0, 400)
pygame.draw.circle(circle_surf, BLACK, (dot_x, dot_y), 10)
# 设置初始角度和距离
angle = math.atan2(dot_y - 200, dot_x - 200)
distance = math.sqrt((dot_x - 200)**2 + (dot_y - 200)**2)
# 程序循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN and event.key == pygame.K_f:
# 计算距离和角度
distance = math.sqrt((dot_x - 200)**2 + (dot_y - 200)**2)
angle = math.atan2(dot_y - 200, dot_x - 200)
# 移动扇形到黑点处
g1_surf.fill(BLACK)
pygame.draw.arc(g1_surf, RED, (0, 0, 400, 400), angle - math.pi/2, angle, 0)
pygame.draw.line(g1_surf, RED, (dot_x, dot_y), (200, 200), 5)
# 绘制圆和扇形
screen.fill(WHITE)
screen.blit(circle_surf, (100, 100))
screen.blit(g1_surf, (100, 100))
# 绘制文字
text1 = font.render("Distance: %.2f" % distance, True, BLACK)
text2 = font.render("Angle: %.2f" % (angle * 180 / math.pi), True, BLACK)
screen.blit(text1, (10, 10))
screen.blit(text2, (10, 40))
# 更新屏幕
pygame.display.flip()
# 退出pygame
pygame.quit()
```
运行程序后,按下F键即可将扇形移动到黑点处,并在控制台输出黑点距离圆心距离和圆心角度。效果如下图所示:

阅读全文
相关推荐
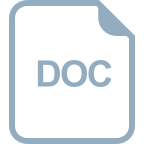
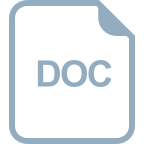
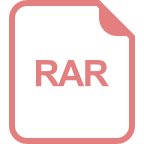
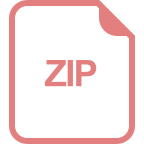
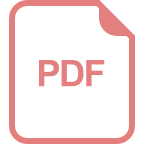
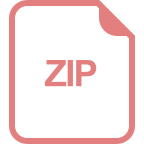
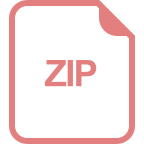
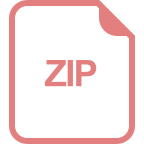
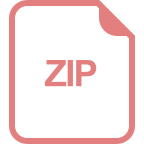
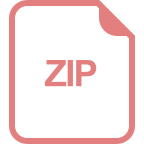
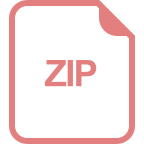
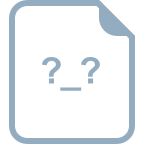
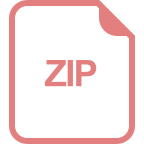
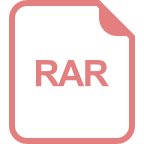