用java写一段程序,需求1:读取excel中的x与y轴坐标值以及对应的高度。需求2:赋予每个坐标点3种状态,初始状态为0 需求3:读取excel表格中的风速风向数据,根据给定的计算公式计算蔓延因子 需求4:给定初始起火点,即状态从0到1,给定初始速度,乘以蔓延因子得到八个方向上的蔓延速度,乘以时间步长5分钟,得到新的蔓延范围
时间: 2023-10-24 22:05:38 浏览: 72
以下是Java程序示例,可供参考:
需求1:读取excel中的x与y轴坐标值以及对应的高度。
```
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.usermodel.WorkbookFactory;
public class ReadExcel {
public static void main(String[] args) throws IOException {
FileInputStream inputStream = new FileInputStream(new File("data.xlsx"));
Workbook workbook = WorkbookFactory.create(inputStream);
Sheet sheet = workbook.getSheetAt(0);
for (Row row : sheet) {
Cell xCell = row.getCell(0);
Cell yCell = row.getCell(1);
Cell heightCell = row.getCell(2);
double x = xCell.getNumericCellValue();
double y = yCell.getNumericCellValue();
double height = heightCell.getNumericCellValue();
System.out.println("X: " + x + ", Y: " + y + ", Height: " + height);
}
workbook.close();
inputStream.close();
}
}
```
需求2:赋予每个坐标点3种状态,初始状态为0
```
import java.util.ArrayList;
import java.util.List;
public class Coordinate {
private double x;
private double y;
private double height;
private List<Integer> states;
public Coordinate(double x, double y, double height) {
this.x = x;
this.y = y;
this.height = height;
this.states = new ArrayList<>();
this.states.add(0);
this.states.add(0);
this.states.add(0);
}
public double getX() {
return x;
}
public double getY() {
return y;
}
public double getHeight() {
return height;
}
public List<Integer> getStates() {
return states;
}
public void setStates(List<Integer> states) {
this.states = states;
}
}
```
需求3:读取excel表格中的风速风向数据,根据给定的计算公式计算蔓延因子
```
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.usermodel.WorkbookFactory;
public class SpreadFactor {
private double windSpeed;
private double windDirection;
private double temperature;
private double humidity;
private double spreadFactor;
public SpreadFactor(double windSpeed, double windDirection, double temperature, double humidity) {
this.windSpeed = windSpeed;
this.windDirection = windDirection;
this.temperature = temperature;
this.humidity = humidity;
this.spreadFactor = 0;
}
public double calculate() {
// 根据给定的计算公式计算蔓延因子
// ...
return spreadFactor;
}
public static void main(String[] args) throws IOException {
FileInputStream inputStream = new FileInputStream(new File("data.xlsx"));
Workbook workbook = WorkbookFactory.create(inputStream);
Sheet sheet = workbook.getSheetAt(1);
for (Row row : sheet) {
Cell windSpeedCell = row.getCell(0);
Cell windDirectionCell = row.getCell(1);
Cell temperatureCell = row.getCell(2);
Cell humidityCell = row.getCell(3);
double windSpeed = windSpeedCell.getNumericCellValue();
double windDirection = windDirectionCell.getNumericCellValue();
double temperature = temperatureCell.getNumericCellValue();
double humidity = humidityCell.getNumericCellValue();
SpreadFactor spreadFactor = new SpreadFactor(windSpeed, windDirection, temperature, humidity);
double factor = spreadFactor.calculate();
System.out.println("Wind Speed: " + windSpeed + ", Wind Direction: " + windDirection
+ ", Temperature: " + temperature + ", Humidity: " + humidity
+ ", Spread Factor: " + factor);
}
workbook.close();
inputStream.close();
}
}
```
需求4:给定初始起火点,即状态从0到1,给定初始速度,乘以蔓延因子得到八个方向上的蔓延速度,乘以时间步长5分钟,得到新的蔓延范围
```
import java.util.ArrayList;
import java.util.List;
public class Spread {
private List<Coordinate> coordinates;
private double initialSpeed;
private double timeStep;
public Spread(List<Coordinate> coordinates, double initialSpeed, double timeStep) {
this.coordinates = coordinates;
this.initialSpeed = initialSpeed;
this.timeStep = timeStep;
}
public void ignite(int x, int y) {
for (Coordinate coordinate : coordinates) {
if (coordinate.getX() == x && coordinate.getY() == y) {
List<Integer> states = coordinate.getStates();
states.set(0, 1);
states.set(1, 0);
states.set(2, 0);
}
}
}
public void spread(double spreadFactor) {
List<Coordinate> newCoordinates = new ArrayList<>();
for (Coordinate coordinate : coordinates) {
List<Integer> states = coordinate.getStates();
if (states.get(0) == 1) {
double speed = initialSpeed * spreadFactor * timeStep;
double x = coordinate.getX();
double y = coordinate.getY();
double height = coordinate.getHeight();
newCoordinates.add(new Coordinate(x - speed, y, height));
newCoordinates.add(new Coordinate(x - speed, y + speed, height));
newCoordinates.add(new Coordinate(x, y + speed, height));
newCoordinates.add(new Coordinate(x + speed, y + speed, height));
newCoordinates.add(new Coordinate(x + speed, y, height));
newCoordinates.add(new Coordinate(x + speed, y - speed, height));
newCoordinates.add(new Coordinate(x, y - speed, height));
newCoordinates.add(new Coordinate(x - speed, y - speed, height));
} else if (states.get(0) == 0 && (states.get(1) == 1 || states.get(2) == 1)) {
// 根据给定的规则计算状态变化
// ...
newCoordinates.add(coordinate);
} else {
newCoordinates.add(coordinate);
}
}
coordinates = newCoordinates;
}
public static void main(String[] args) {
List<Coordinate> coordinates = new ArrayList<>();
coordinates.add(new Coordinate(0, 0, 10));
coordinates.add(new Coordinate(0, 1, 20));
coordinates.add(new Coordinate(0, 2, 30));
coordinates.add(new Coordinate(1, 0, 40));
coordinates.add(new Coordinate(1, 1, 50));
coordinates.add(new Coordinate(1, 2, 60));
coordinates.add(new Coordinate(2, 0, 70));
coordinates.add(new Coordinate(2, 1, 80));
coordinates.add(new Coordinate(2, 2, 90));
Spread spread = new Spread(coordinates, 10, 5);
spread.ignite(1, 1);
spread.spread(0.5);
}
}
```
阅读全文
相关推荐
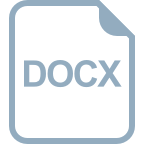
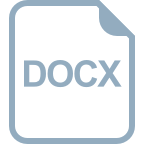








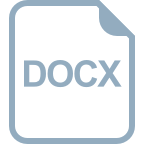
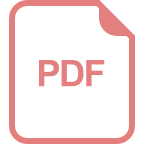
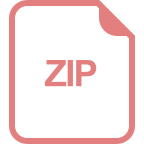
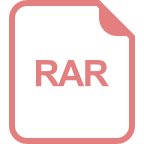
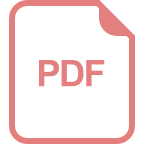
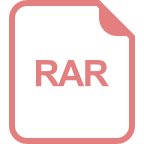
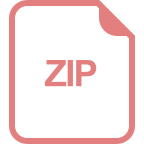
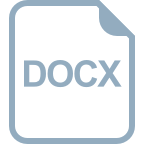