string StringHandler::removeDuplicateLetters(string s) { for (char c : s) { count[c - 'a']++; } for (char c : s) { count[c - 'a']--; if (inStack[c - 'a']) { continue; } while (!st.empty() && st.top() > c && count[st.top() - 'a'] > 0) { inStack[st.top() - 'a'] = false; st.pop(); } st.push(c); inStack[c - 'a'] = true; } string ans = ""; while (!st.empty()) { ans += st.top(); st.pop(); } reverse(ans.begin(), ans.end()); return ans; }解释每一行代码
时间: 2023-12-14 20:38:37 浏览: 18
这段代码实现了去除字符串中重复字符的功能,下面是每一行代码的详细解释:
```c++
string StringHandler::removeDuplicateLetters(string s) {
```
定义一个名为 `removeDuplicateLetters` 的函数,返回值类型为 `string`,参数类型为 `string`,参数名为 `s`。
```c++
for (char c : s) {
count[c - 'a']++;
}
```
遍历字符串 `s` 中的每一个字符,并记录其出现次数。使用一个大小为 `26` 的数组 `count` 来记录,`count[i]` 表示字符 `'a' + i'` 出现的次数。
```c++
for (char c : s) {
count[c - 'a']--;
if (inStack[c - 'a']) {
continue;
}
while (!st.empty() && st.top() > c && count[st.top() - 'a'] > 0) {
inStack[st.top() - 'a'] = false;
st.pop();
}
st.push(c);
inStack[c - 'a'] = true;
}
```
再次遍历字符串 `s` 中的每一个字符,并把每个字符加入到一个栈中。同时,用一个 `bool` 类型的数组 `inStack` 来记录每个字符是否已经在栈中,避免同一个字符被加入到栈中多次。
对于每个字符 `c`,先把 `count[c - 'a']` 减一,表示这个字符已经被加入到栈中。如果这个字符 `c` 已经在栈中,那么直接跳过。否则,我们需要把栈中比当前字符 `c` 大且后面还会出现的字符弹出,避免后面的更小的字符被这些大的字符挡住。
```c++
string ans = "";
while (!st.empty()) {
ans += st.top();
st.pop();
}
reverse(ans.begin(), ans.end());
return ans;
}
```
将栈中剩余的字符倒序输出,得到去重后的字符串,并返回结果。
相关推荐
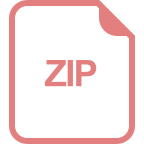
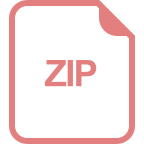
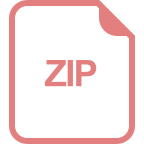
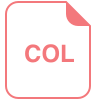
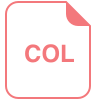
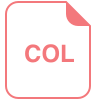
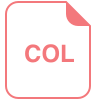
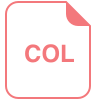









