解释代码:string = input("Enter a string: ")char_count = {}for char in string: if char in char_count: char_count[char] += 1 else: char_count[char] = 1for char, count in char_count.items(): print(char, count)
时间: 2023-05-13 15:07:08 浏览: 100
这段代码是一个 Python 程序,它的作用是统计一个字符串中每个字符出现的次数,并输出结果。首先,程序会要求用户输入一个字符串,然后定义一个空字典 char_count 用于存储每个字符出现的次数。接着,程序会遍历字符串中的每个字符,如果该字符已经在 char_count 中出现过,则将其出现次数加 1,否则将其加入 char_count 中,并将其出现次数设为 1。最后,程序会遍历 char_count 中的每个键值对,输出每个字符和其出现次数。
相关问题
Write a program that takes a string input from the user and counts the number of unique characters in the string. Note:The program should only count each unique character once, even if it appears multiple times i n the input string. The program should als
o ignore spaces and special characters.
Sure, here's a Python program that does what you asked:
```
def count_unique_chars(string):
unique_chars = set()
for char in string:
if char.isalnum():
unique_chars.add(char.lower())
return len(unique_chars)
user_input = input("Enter a string: ")
print("Number of unique characters:", count_unique_chars(user_input))
```
Explanation:
- The `count_unique_chars` function takes a string as input and returns the number of unique characters in it.
- We create an empty set called `unique_chars` to keep track of unique characters as we iterate through the string.
- For each character in the string, we check if it is alphanumeric (i.e., a letter or a number), and convert it to lowercase using the `lower()` method. If it is, we add it to the `unique_chars` set.
- Finally, we return the length of the `unique_chars` set, which gives us the number of unique characters in the string.
- In the main program, we prompt the user to enter a string, and then call the `count_unique_chars` function on it. The result is printed to the console.
I hope this helps! Let me know if you have any further questions.
3. Calculate the number of characters: write a program to receive a character string and a character composed of letters, numbers and spaces, and then output the number of characters contained in the input character string, regardless of case. Input: ABAOP123 A. Output: 2. 4. Enter a line of characters and count the numbers of English letters, spaces, numbers and other characters.
Here are two programs - one for calculating the number of a specific character in a string, and another for counting the number of different types of characters in a string:
Program 1: Counting the number of a specific character in a string
```python
string = input("Enter a string: ")
char = input("Enter a character: ")
count = 0
for c in string:
if c.lower() == char.lower():
count += 1
print(f"The string '{string}' contains {count} instances of the character '{char}'.")
```
Program 2: Counting the number of different types of characters in a string
```python
string = input("Enter a string: ")
num_letters = 0
num_spaces = 0
num_numbers = 0
num_other = 0
for c in string:
if c.isalpha():
num_letters += 1
elif c.isspace():
num_spaces += 1
elif c.isdigit():
num_numbers += 1
else:
num_other += 1
print(f"The string '{string}' contains:")
print(f"- {num_letters} letters")
print(f"- {num_spaces} spaces")
print(f"- {num_numbers} numbers")
print(f"- {num_other} other characters")
```
阅读全文
相关推荐
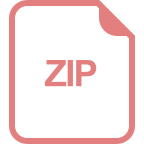
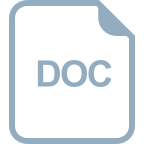
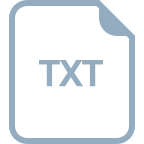













