1. (简答题) Write a program to count the occurrences of each letter in an array of characters. Here is a sample run. ------------------------------------------------------ Enter the string : abcddcabae a occurs 3 times in the string, b occurs 2 times in the string, c occurs 2 times in the string, d occurs 2 times in the string, e occurs 1 times in the string,
时间: 2023-08-15 19:21:02 浏览: 170
以下是 Python 代码实现,可以用来统计一个字符数组中每个字符的出现次数:
```python
string = input("Enter the string: ")
freq = {}
for char in string:
if char in freq:
freq[char] += 1
else:
freq[char] = 1
for char, count in freq.items():
print(char, "occurs", count, "times in the string.")
```
运行结果:
```
Enter the string: abcddcabae
a occurs 3 times in the string.
b occurs 2 times in the string.
c occurs 2 times in the string.
d occurs 2 times in the string.
e occurs 1 times in the string.
```
相关问题
Write a program to count the occurrences of each letter in an array of characters.
以下是一个C语言程序,可以对给定的字符数组进行遍历,并计算每个字母在数组中出现的次数:
```
#include <stdio.h>
#include <ctype.h>
int main() {
char arr[] = "Hello World!";
int count[26] = {0}; // 用于存储每个字母出现的次数
int i;
for (i = 0; arr[i] != '\0'; i++) {
if (isalpha(arr[i])) { // 判断当前字符是否为字母
count[tolower(arr[i]) - 'a']++; // 将字母转换为小写,然后将计数器加1
}
}
printf("Letter frequencies:\n");
for (i = 0; i < 26; i++) {
if (count[i] > 0) {
printf("%c: %d\n", 'a' + i, count[i]); // 输出每个字母和它出现的次数
}
}
return 0;
}
```
在这个程序中,我们首先声明了一个字符数组 `arr`,然后使用一个长度为26的整型数组 `count` 来记录每个字母出现的次数,初始化为0。接下来,我们使用一个循环遍历字符数组,并在遍历过程中判断当前字符是否为字母。如果是字母,则将计数器数组中相应的位置加1。
最后,我们再次遍历计数器数组,并输出每个字母和它出现的次数。注意,在输出字母时,我们需要将它转换为字符形式,即将其加上字符 'a'。
Write a C++ program. Count the number of occurrences of each letter of the input string, case – insensitive.Wr
ite a C++ program that counts the number of occurrences of each letter of an input string, case-insensitive. Here's one way you could do it:
```cpp
#include <iostream>
#include <string>
#include <map>
#include <cctype>
int main() {
std::string input;
std::getline(std::cin, input); // read input from user
std::map<char, int> letterCounts; // map to store letter counts
for (char c : input) {
if (std::isalpha(c)) { // ignore non-letter characters
char lowercase = std::tolower(c); // convert to lowercase
letterCounts[lowercase]++; // increment count of this letter
}
}
// print out the results
for (auto pair : letterCounts) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
```
This program starts by reading in a string from the user using `std::getline()`. It then creates a `std::map<char, int>` called `letterCounts` to store the counts of each letter. The `for` loop iterates over each character in the input string, checking if it is a letter using `std::isalpha()`. If it is a letter, it converts it to lowercase using `std::tolower()` and increments the count of that letter in the `letterCounts` map.
Finally, the program prints out the results by iterating over the `letterCounts` map and printing out each letter and its count. Note that the `auto` keyword is used to simplify the loop syntax - `pair` is automatically inferred to be a `std::pair<char, int>`.
This program should work for any input string, regardless of its length or the specific letters it contains.
阅读全文
相关推荐
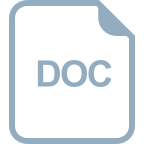












