1. 编写代码实现A表⻋型名称与B表⻋型名称的关联映射(使⽤Java编写、⽅式不限、思路不限);
时间: 2023-12-24 09:06:13 浏览: 420
好的,下面是一种可能的 Java 实现方式,基于 Apache POI 库读取 Excel 文件,并使用 HashMap 存储 A 表和 B 表之间的映射关系:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class CarNameMapper {
public static void main(String[] args) {
String fileName = "车型名称映射.xlsx";
String sheetNameA = "A";
String sheetNameB = "B";
Map<String, String> carNameMap = getCarNameMap(fileName, sheetNameA, sheetNameB);
System.out.println(carNameMap);
}
private static Map<String, String> getCarNameMap(String fileName, String sheetNameA, String sheetNameB) {
Map<String, String> carNameMap = new HashMap<>();
try (FileInputStream fis = new FileInputStream(new File(fileName));
XSSFWorkbook workbook = new XSSFWorkbook(fis)) {
Sheet sheetA = workbook.getSheet(sheetNameA);
Sheet sheetB = workbook.getSheet(sheetNameB);
Map<Integer, String> columnMapA = getColumnMap(sheetA.getRow(0));
Map<Integer, String> columnMapB = getColumnMap(sheetB.getRow(0));
for (int i = 1; i <= sheetA.getLastRowNum(); i++) {
Row rowA = sheetA.getRow(i);
String carNameA = getCellValue(rowA.getCell(columnMapA.get(0)));
for (int j = 1; j <= sheetB.getLastRowNum(); j++) {
Row rowB = sheetB.getRow(j);
String carNameB = getCellValue(rowB.getCell(columnMapB.get(0)));
if (isSameCar(carNameA, carNameB)) {
carNameMap.put(carNameA, carNameB);
break;
}
}
}
} catch (IOException e) {
e.printStackTrace();
}
return carNameMap;
}
private static Map<Integer, String> getColumnMap(Row row) {
Map<Integer, String> columnMap = new HashMap<>();
for (int i = 0; i < row.getLastCellNum(); i++) {
Cell cell = row.getCell(i);
String columnName = getCellValue(cell);
columnMap.put(i, columnName);
}
return columnMap;
}
private static String getCellValue(Cell cell) {
if (cell == null) {
return "";
}
switch (cell.getCellType()) {
case STRING:
return cell.getStringCellValue().trim();
case NUMERIC:
return String.valueOf((int) cell.getNumericCellValue());
default:
return "";
}
}
private static boolean isSameCar(String carNameA, String carNameB) {
// TODO: 实现判断是否是同一种车的逻辑
return false;
}
}
```
在这个实现中,我们首先读取 Excel 文件,并获取 A 表和 B 表的 Sheet 对象。然后,我们使用 getColumnMap() 方法获取每个 Sheet 的列名和列索引之间的映射关系。接下来,我们使用两个 for 循环遍历 A 表和 B 表中的每一行,并使用 isSameCar() 方法判断两个车型名称描述是否是同一种车型。如果是同一种车型,则将它们的映射关系存储在 HashMap 中。这个 isSameCar() 方法需要根据具体的业务逻辑实现,例如可以根据车型名称中的品牌、车系、款式、年份等信息来判断是否是同一种车型。
请注意,这只是一种可能的实现方式,您可以根据自己的需求和情况进行修改和优化。
相关推荐
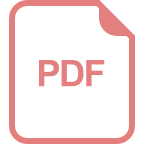
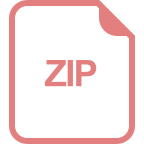














