安卓环境onvif 协议读取视频流并提取每一帧图像的代码
时间: 2023-06-16 20:06:12 浏览: 76
以下是一个简单的示例代码,用于从 ONVIF 设备读取视频流并提取每一帧图像:
```java
import javax.xml.soap.SOAPException;
import javax.xml.ws.soap.SOAPFaultException;
import org.onvif.ver10.schema.VideoEncoderConfiguration;
import org.onvif.ver10.schema.VideoResolution;
import org.onvif.ver10.schema.VideoSource;
import org.onvif.ver10.schema.VideoSourceConfiguration;
import com.sun.xml.ws.client.ClientTransportException;
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.Image;
import java.awt.image.BufferedImage;
import java.io.*;
import java.net.ConnectException;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.ArrayList;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import javax.imageio.ImageIO;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class ONVIFExample extends JPanel {
private static final long serialVersionUID = 1L;
private static final String MEDIA_PROFILE_TOKEN = "MediaProfile000";
private static final String SERVICE_URI = "http://192.168.1.1/onvif/device_service";
private static final String USER = "admin";
private static final String PASSWORD = "admin";
private BufferedImage currentImage = null;
public ONVIFExample() {
super();
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
if (currentImage != null) {
g.drawImage(currentImage, 0, 0, this);
}
}
public void readStream() throws Exception {
String videoUrl = getVideoUrl(SERVICE_URI, USER, PASSWORD);
if (videoUrl == null) {
throw new Exception("Failed to get video URL");
}
String[] urlParts = videoUrl.split(":");
String host = urlParts[0];
int port = Integer.parseInt(urlParts[1]);
InputStream inputStream = null;
ByteArrayOutputStream byteArrayOutputStream = null;
try {
URL url = new URL(videoUrl);
HttpURLConnection httpConn = (HttpURLConnection) url.openConnection();
httpConn.setRequestProperty("Authorization", "Basic " + getEncodedAuth(USER, PASSWORD));
inputStream = httpConn.getInputStream();
byteArrayOutputStream = new ByteArrayOutputStream();
byte[] chunk = new byte[4096];
int bytesRead;
while ((bytesRead = inputStream.read(chunk)) > 0) {
byteArrayOutputStream.write(chunk, 0, bytesRead);
if (byteArrayOutputStream.size() > 1024 * 1024) {
currentImage = getBufferedImage(byteArrayOutputStream.toByteArray());
byteArrayOutputStream.reset();
repaint();
}
}
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
if (inputStream != null) {
try {
inputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (byteArrayOutputStream != null) {
try {
byteArrayOutputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
private static String getVideoUrl(String serviceUrl, String user, String pass) {
String mediaUrl = null;
try {
DeviceService deviceService = new DeviceService(serviceUrl, user, pass);
VideoEncoderConfiguration encoderConfiguration = deviceService.getVideoEncoderConfiguration(MEDIA_PROFILE_TOKEN);
VideoSourceConfiguration sourceConfiguration = deviceService.getVideoSourceConfiguration(encoderConfiguration.getSourceToken());
VideoSource videoSource = deviceService.getVideoSource(sourceConfiguration.getSourceToken());
VideoResolution resolution = encoderConfiguration.getResolution();
Dimension dimension = new Dimension(resolution.getWidth(), resolution.getHeight());
mediaUrl = deviceService.getSnapshotUri(dimension.width, dimension.height);
} catch (SOAPFaultException e) {
e.printStackTrace();
} catch (ConnectException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} catch (SOAPException e) {
e.printStackTrace();
}
return mediaUrl;
}
private static String getEncodedAuth(String user, String pass) {
String authStr = user + ":" + pass;
String authEncoded = javax.xml.bind.DatatypeConverter.printBase64Binary(authStr.getBytes());
return authEncoded;
}
private static BufferedImage getBufferedImage(byte[] imageData) {
BufferedImage bufferedImage = null;
try {
InputStream inputStream = new ByteArrayInputStream(imageData);
bufferedImage = ImageIO.read(inputStream);
} catch (IOException e) {
e.printStackTrace();
}
return bufferedImage;
}
public static void main(String[] args) {
JFrame frame = new JFrame("ONVIF Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
ONVIFExample onvifExample = new ONVIFExample();
frame.add(onvifExample);
frame.setSize(640, 480);
frame.setVisible(true);
try {
onvifExample.readStream();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
这个示例代码使用了 ONVIF 的 Java 实现,它可以帮助你通过 ONVIF 协议从相应的设备获取视频流。在获得视频流之后,代码会将每一帧图像转换为 BufferedImage,并在 JPanel 上绘制出来。你可以根据你的需求修改代码以实现更多功能。
相关推荐
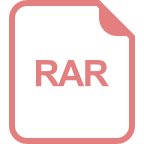
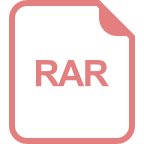














