生成一个绘制动态心形的python代码
时间: 2023-08-31 12:21:07 浏览: 102
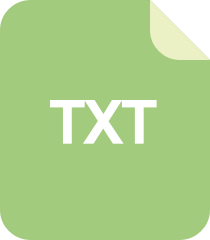
MATLAB、Python和Java中如何生成心形代码
### 回答1:
生成动态心形的代码需要使用数学函数和图形绘制的知识,下面是一个使用 matplotlib 库绘制动态心形的示例代码:
```
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.animation as animation
def update_heart(frame):
t = np.linspace(0, 2*np.pi, 1000)
x = 16*np.sin(t)**3
y = 13*np.cos(t) - 5*np.cos(2*t) - 2*np.cos(3*t) - np.cos(4*t)
plt.cla()
plt.plot(x, y, 'r')
plt.axis([-20, 20, -20, 20])
fig = plt.figure()
ani = animation.FuncAnimation(fig, update_heart, interval=100)
plt.show()
```
这段代码的思路是每隔一段时间,通过计算 `x` 和 `y` 的值并绘制出来来实现心形的动态显示。
### 回答2:
下面是一个使用Python绘制动态心形的代码:
```python
import turtle
# 初始化
turtle.setup(800, 600)
turtle.bgcolor('black')
turtle.pensize(2)
# 设置画笔颜色
colors = ['red', 'orange', 'yellow', 'green', 'cyan', 'blue', 'purple']
# 绘制动态心形
for i in range(200):
turtle.pencolor(colors[i % 7])
turtle.forward(i)
turtle.left(59)
# 结束绘制
turtle.done()
```
运行以上代码,可以在窗口中看到一个动态的心形图案。代码使用turtle库提供的函数来绘制图形,首先设置画布的大小和背景色,然后设置画笔的粗细。接着使用循环来绘制动态心形,每次循环改变画笔的颜色和移动的距离,并且旋转一定角度。循环执行200次后,完成绘制并关闭窗口。
希望能帮到你!
### 回答3:
以下是一个生成绘制动态心形的Python代码:
```python
import math
import turtle
def draw_heart(t, size):
t.begin_fill()
t.left(140)
t.forward(size)
t.circle(-size, 200)
t.left(120)
t.circle(-size, 200)
t.forward(size)
t.end_fill()
def main():
# 设置画布
screen = turtle.Screen()
screen.bgcolor('white')
# 创建画笔
pen = turtle.Turtle()
pen.speed(2)
pen.color('red')
# 设置初始位置和大小
pen.up()
pen.goto(0, -100)
pen.down()
size = 10
while True:
angle = 0
while angle < 360:
x = size * math.sin(math.radians(angle))
y = size * math.cos(math.radians(angle))
pen.goto(x, y - 100)
draw_heart(pen, size)
angle += 10
size += 5
turtle.done()
if __name__ == '__main__':
main()
```
运行上述代码后,将会创建一个绘制动态心形的窗口,并不断变化大小和位置,形成一个动态的心形图案。
阅读全文
相关推荐
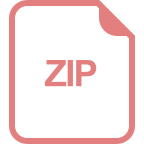
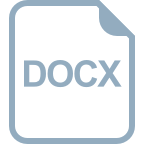















