C++用二帮我写一个C++程序用二叉树将文本文档中的字符进行霍夫曼编码
时间: 2023-09-11 07:07:44 浏览: 132
好的,下面是一个简单的C++程序,使用二叉树将文本文档中的字符进行霍夫曼编码:
```c++
#include <iostream>
#include <fstream>
#include <queue>
#include <unordered_map>
#include <bitset>
using namespace std;
//定义霍夫曼树的节点结构体
struct HuffmanNode {
char ch; //字符
int freq; //出现频率
HuffmanNode *left; //左子节点
HuffmanNode *right; //右子节点
HuffmanNode(char ch, int freq, HuffmanNode *left = nullptr, HuffmanNode *right = nullptr) {
this->ch = ch;
this->freq = freq;
this->left = left;
this->right = right;
}
};
//定义优先队列的比较函数
struct Compare {
bool operator()(HuffmanNode *a, HuffmanNode *b) {
return a->freq > b->freq;
}
};
//统计文本文档中各个字符出现的频率
unordered_map<char, int> getFreqTable(string filename) {
unordered_map<char, int> freq_table;
ifstream infile(filename);
char ch;
while (infile >> noskipws >> ch) {
freq_table[ch]++;
}
infile.close();
return freq_table;
}
//根据频率表构建霍夫曼树
HuffmanNode *buildHuffmanTree(unordered_map<char, int> freq_table) {
priority_queue<HuffmanNode *, vector<HuffmanNode *>, Compare> pq;
for (auto it = freq_table.begin(); it != freq_table.end(); it++) {
pq.push(new HuffmanNode(it->first, it->second));
}
while (pq.size() > 1) {
HuffmanNode *node1 = pq.top();
pq.pop();
HuffmanNode *node2 = pq.top();
pq.pop();
pq.push(new HuffmanNode('\0', node1->freq + node2->freq, node1, node2));
}
return pq.top();
}
//遍历霍夫曼树,生成每个字符的编码
void generateCodeTable(HuffmanNode *root, unordered_map<char, string> &code_table, string code = "") {
if (root == nullptr) {
return;
}
if (root->left == nullptr && root->right == nullptr) {
code_table[root->ch] = code;
}
generateCodeTable(root->left, code_table, code + "0");
generateCodeTable(root->right, code_table, code + "1");
}
//将文本文档中的每个字符替换为其对应的霍夫曼编码,并将编码后的文本存储到一个新的文件中
void compressFile(string input_filename, string output_filename, unordered_map<char, string> code_table) {
ifstream infile(input_filename);
ofstream outfile(output_filename);
char ch;
string code;
while (infile >> noskipws >> ch) {
code += code_table[ch];
while (code.size() >= 8) {
bitset<8> b(code.substr(0, 8));
code = code.substr(8);
outfile << (char)b.to_ulong();
}
}
if (code.size() > 0) {
bitset<8> b(code + string(8 - code.size(), '0'));
outfile << (char)b.to_ulong();
}
infile.close();
outfile.close();
}
//解码函数,将编码后的文本解码为原始文本
void decompressFile(string input_filename, string output_filename, HuffmanNode *root) {
ifstream infile(input_filename);
ofstream outfile(output_filename);
HuffmanNode *cur = root;
char ch;
while (infile >> noskipws >> ch) {
bitset<8> b(ch);
for (int i = 7; i >= 0; i--) {
if (b[i] == 0) {
cur = cur->left;
} else {
cur = cur->right;
}
if (cur->left == nullptr && cur->right == nullptr) {
outfile << cur->ch;
cur = root;
}
}
}
infile.close();
outfile.close();
}
int main() {
string input_filename = "input.txt";
string output_filename = "output.bin";
string decompressed_filename = "decompressed.txt";
unordered_map<char, int> freq_table = getFreqTable(input_filename);
HuffmanNode *root = buildHuffmanTree(freq_table);
unordered_map<char, string> code_table;
generateCodeTable(root, code_table);
compressFile(input_filename, output_filename, code_table);
decompressFile(output_filename, decompressed_filename, root);
return 0;
}
```
这个程序会读取名为"input.txt"的文本文档,并将其中的字符进行霍夫曼编码,将编码后的结果存储到名为"output.bin"的文件中。然后,程序会将"output.bin"文件中的编码解码为原始文本,并将结果存储到名为"decompressed.txt"的文件中。
阅读全文
相关推荐


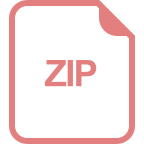





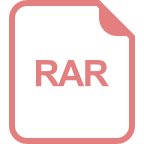
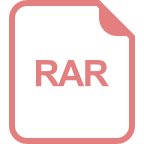
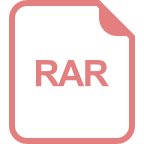




