房间内人群疏散的python简单模拟
时间: 2023-10-19 09:36:26 浏览: 110
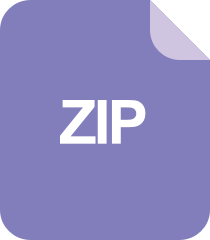
基于Python模拟SFM社会力模型单房间疏散场景【100012953】

对于人群疏散的简单模拟,你可以使用 Python 来实现。以下是一个可能的实现示例:
```python
import random
class Person:
def __init__(self, x, y):
self.x = x
self.y = y
def move(self):
direction = random.choice(['up', 'down', 'left', 'right'])
if direction == 'up':
self.y -= 1
elif direction == 'down':
self.y += 1
elif direction == 'left':
self.x -= 1
elif direction == 'right':
self.x += 1
class Room:
def __init__(self, width, height):
self.width = width
self.height = height
self.people = []
def add_person(self, x, y):
person = Person(x, y)
self.people.append(person)
def simulate(self, steps):
for step in range(steps):
for person in self.people:
person.move()
# 检查边界
person.x = max(0, min(person.x, self.width - 1))
person.y = max(0, min(person.y, self.height - 1))
def display(self):
for y in range(self.height):
for x in range(self.width):
if any(person.x == x and person.y == y for person in self.people):
print('X', end=' ')
else:
print('_', end=' ')
print()
# 创建一个房间对象
room = Room(10, 10)
# 在房间中添加人员
room.add_person(5, 5)
room.add_person(7, 8)
# 模拟人员移动
room.simulate(10)
# 显示房间状态
room.display()
```
这个简单的模拟程序中,首先定义了一个 `Person` 类来表示每个人的位置,`Room` 类用于管理房间和人员。`simulate` 方法用于模拟人员的移动,`display` 方法用于显示当前房间状态。你可以根据需要修改房间的大小、添加更多的人员,以及调整模拟的步数。运行这段代码后,你会看到模拟后的房间状态以及人员的移动路径。
阅读全文
相关推荐

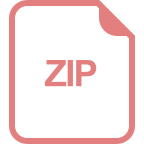
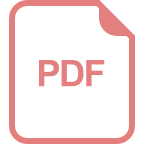
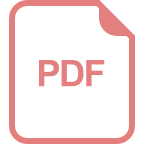
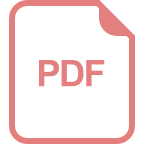
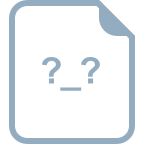
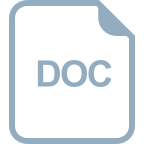
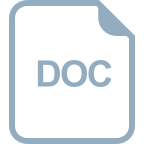
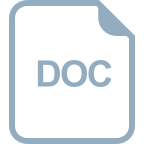
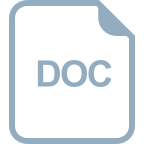



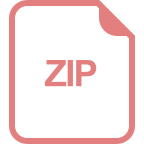