完成这个c++编程题
时间: 2024-12-05 20:21:29 浏览: 20
为了帮助你完成这个C++编程任务,我将提供一个详细的步骤指南以及关键代码片段。这个任务的主要目标是从命令行读取参数,并将其转换为会计数字的形式。
### 步骤指南
1. **读取命令行参数**:
- 使用 `main` 函数的 `argc` 和 `argv` 参数来读取命令行参数。
- `argc` 表示参数的数量,`argv` 是一个字符串数组,其中 `argv[0]` 是程序名称,其他元素是传入的参数。
2. **处理每个参数**:
- 对每个参数进行解析,判断其是否有小数部分。
- 将数字字符串转换为整数和小数部分。
3. **转换为会计数字**:
- 使用数组来存储数字 0-9 的中文大写形式。
- 处理整数部分和小数部分,生成相应的会计数字字符串。
4. **输出结果**:
- 对于每个参数,输出其对应的会计数字形式。
### 关键代码片段
#### 主函数
```cpp
#include <iostream>
#include <cstdlib>
#include <cstring>
using namespace std;
// 数字到中文大写的映射
const char* digitToChinese[] = {"零", "壹", "贰", "叁", "肆", "伍", "陆", "柒", "捌", "玖"};
const char* unitSmall[] = {"元", "角", "分"};
const char* unitBig[] = {"", "拾", "佰", "仟", "万", "拾", "佰", "仟", "亿"};
string toChineseNum(const char* numStr) {
// 实现将数字字符串转换为会计数字的逻辑
}
int main(int argc, char* argv[]) {
if (argc <= 1) {
cout << "No input parameters provided." << endl;
return 1;
}
for (int i = 1; i < argc; ++i) {
string result = toChineseNum(argv[i]);
cout << result << endl;
}
return 0;
}
```
#### 转换函数
```cpp
string toChineseNum(const char* numStr) {
char* end;
long long integerPart = strtoll(numStr, &end, 10);
double fractionalPart = 0.0;
bool hasFractional = false;
if (*end == '.') {
fractionalPart = strtod(end, nullptr);
hasFractional = true;
}
string result;
// 处理整数部分
if (integerPart == 0) {
result += "零元";
} else {
bool leadingZero = false;
bool isZero = false;
int index = 0;
while (integerPart > 0) {
int digit = integerPart % 10;
integerPart /= 10;
if (digit == 0) {
if (!isZero && index != 0) {
result.insert(0, digitToChinese[digit]);
isZero = true;
}
} else {
if (isZero) {
result.insert(0, "零");
isZero = false;
}
result.insert(0, unitBig[index]);
result.insert(0, digitToChinese[digit]);
}
if (index == 3 || index == 7) { // 万、亿
result.insert(0, unitBig[index + 1]);
}
index++;
}
result += "元";
}
// 处理小数部分
if (hasFractional) {
int fractionInt = static_cast<int>(fractionalPart * 100 + 0.5); // 四舍五入
if (fractionInt > 0) {
int jiao = fractionInt / 10;
int fen = fractionInt % 10;
if (jiao > 0) {
result += digitToChinese[jiao];
result += "角";
}
if (fen > 0) {
result += digitToChinese[fen];
result += "分";
}
} else {
result += "整";
}
} else {
result += "整";
}
return result;
}
```
### 编译和运行
1. **编译**:
```sh
g++ --std=c++11 your_program.cpp -o your_program -fexec-charset=GBK -finput-charset=UTF-8
```
2. **运行**:
```sh
./your_program 10.0 1000 100002 532.00 425.03 7510.31 289546.52 101.50 1004.56 10000.1 1010100101.01 2147483647.99
```
### 注意事项
- 确保你的代码能够处理各种边界情况,如全零、带小数的零等。
- 使用 `strtoll` 和 `strtod` 来处理整数和小数部分的转换。
- 处理中文字符编码问题,确保输出不会出现乱码。
通过上述步骤和代码片段,你应该能够完成这个C++编程任务。如果有任何问题或需要进一步的帮助,请随时告诉我。
阅读全文
相关推荐







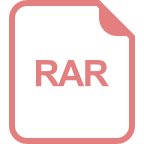




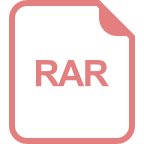





