string能直接比较排序吗c++
时间: 2024-11-24 09:40:38 浏览: 15
在C++中,`std::string` 类型的对象可以使用 `<`, `<=`, `>=`, 和 `>` 运算符进行比较,但这实际上是基于它们的字典顺序[^1],即按照字符编码顺序进行比较。如果你想要自定义排序规则,你可以创建一个比较函数(如 `cmp()` 函数示例[^2]),该函数接收两个 `string` 参数并返回一个布尔值,表示第一个参数是否应该排在第二个参数之前。
例如,为了对字符串进行升序排序,你可以这样实现:
```cpp
#include <algorithm>
#include <vector>
// 自定义比较函数
bool myCompare(const std::string& a, const std::string& b) {
return a.compare(b) < 0;
}
int main() {
std::vector<std::string> vec = {"banana", "apple", "cherry"};
std::sort(vec.begin(), vec.end(), myCompare); // 使用自定义比较函数
for (const auto& s : vec) {
std::cout << s << " ";
}
return 0;
}
```
运行这段代码会得到按字母升序排列的字符串序列。
相关问题
string 能自排序吗,c++
在C++中,`std::string` 类型本身并不支持直接的自排序,因为它们是不可比较的对象。但是,你可以通过使用`<algorithm>`库中的`sort()`函数,配合自定义的比较函数来对存储字符串的数组进行排序。
**示例**:
```cpp
#include <iostream>
#include <string>
#include <algorithm> // 引入sort函数
using namespace std;
// 自定义比较函数,用于按字母顺序比较字符串
bool cmp(string str1, string str2) {
return str1 < str2;
}
int main() {
string a[6];
for (int i = 0; i < 4; i++) {
getline(cin, a[i]);
}
// 使用sort和cmp函数按字母顺序排序
sort(a, a + 4, cmp);
for (int i = 0; i < 4; i++) {
cout << a[i] << "\n"; // 注意这里不需要.c_str()
}
return 0;
}
```
在这个例子中,`getline()`从标准输入读取字符串到数组`a`,然后`sort()`函数会按照`cmp`函数的逻辑对这些字符串进行升序排列。如果你想要降序排列,只需改变`cmp`函数:
```cpp
bool cmp(string str1, string str2) {
return str1 > str2;
}
```
string类的字符串排序c++
可以使用`sort`函数对字符串数组排序,示例代码如下:
```c++
#include <iostream>
#include <algorithm>
#include <string>
using namespace std;
int main() {
string arr[] = {"apple", "banana", "orange", "grape"};
int n = sizeof(arr) / sizeof(arr[0]);
sort(arr, arr + n);
for (int i = 0; i < n; i++) {
cout << arr[i] << " ";
}
cout << endl;
return 0;
}
```
上述代码中,我们定义了一个字符串数组`arr`,然后使用`sort`函数对其进行排序。最后遍历数组并输出排序结果。需要注意的是,我们在`sort`函数中传入的第二个参数是指向数组末尾的迭代器,而不是数组长度。
阅读全文
相关推荐


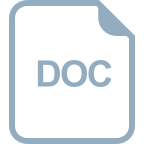













