Visual求100以内的素数
时间: 2024-12-19 14:21:46 浏览: 5
Visual Studio并不是直接用于计算素数的工具,它是一个集成开发环境(IDE),主要用于编写各种应用程序,包括Windows应用、网站等。然而,如果你想要用Visual Studio编写一个小程序来找出100以内的素数,你可以这样做:
1. 创建一个新的控制台应用项目。
2. 使用循环和条件判断来遍历1到100之间的整数。
3. 对每个数检查是否能被2到其平方根之间的任意整数整除,如果都不能,则它是素数。
4. 输出找到的所有素数。
下面是一个简单的伪代码示例:
```vb.net
For i As Integer = 2 To 100
IsPrime = True
For j As Integer = 2 To Math.Sqrt(i)
If i Mod j = 0 Then
IsPrime = False
Exit For ' 如果找到因子就退出内层循环
End If
Next
If IsPrime Then
Console.WriteLine(i) ' 打印素数
End If
Next
```
运行这个程序后,你会看到控制台上显示所有100以内的素数。
相关问题
使用visual Studio打印出100以内所有质数有多少个
Visual Studio是一款强大的集成开发环境,用于多种编程语言,包括C#。要在Visual Studio中编写代码来找出100以内所有的质数,你可以使用C#编写一个简单的算法程序。以下是一个示例:
```csharp
using System;
class Program
{
static void Main()
{
int count = 0; // 计数器,记录质数个数
for (int i = 2; i <= 100; i++)
{
if (IsPrime(i)) // 判断是否为质数
{
Console.WriteLine($"Number {i} is prime."); // 打印质数
count++; // 当找到质数时,计数器加一
}
}
Console.WriteLine($"{count} prime numbers are less than or equal to 100."); // 输出总共有多少个质数
}
// 辅助函数:判断一个数是否为质数
static bool IsPrime(int num)
{
if (num < 2) return false;
for (int j = 2; j * j <= num; j++) // 遍历到其平方根即可
{
if (num % j == 0) // 如果能整除,则不是质数
return false;
}
return true;
}
}
```
在这个程序中,我们遍历从2到100的所有数字,通过`IsPrime`函数检查每个数字是否是质数。如果是,就打印出来并增加计数器。最后,程序会显示小于等于100的质数总数。
写出一段代码在visual basic 筛选出1000以内质数
很高兴为您提供帮助。以下是Visual Basic中筛选出1000以内质数的代码:
Private Sub btnPrimeNumbers_Click()
Dim count As Integer
Dim i As Integer
Dim j As Integer
Dim isPrime As Boolean
lstPrimeNumbers.Clear
'finding prime numbers up to 1000
For i = 2 To 1000
isPrime = True
For j = 2 To i - 1
If i Mod j = 0 Then 'if the number is divisible by any number other than itself or 1
isPrime = False
Exit For
End If
Next j
If isPrime Then
lstPrimeNumbers.AddItem i 'add the prime number to list box
count = count + 1 'increment the counter
End If
Next i
'displaying the count of prime numbers found
MsgBox count & " prime numbers found."
End Sub
请注意,这段代码只能筛选出1000以内的质数。如果您需要寻找更大的质数,请相应地更改代码。同时,这段代码是通过遍历每个整数来查找质数,因此处理较大的数字时可能需要更长的时间。
阅读全文
相关推荐














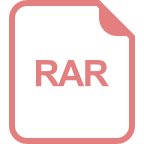

