能不能用python脚本抓取.log文件里的数据存放到excel表格中
时间: 2024-02-19 09:03:35 浏览: 146
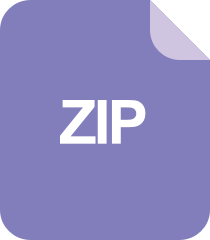
changelog-gentoo:python脚本为包生成类似changelog的输出
可以的,你可以使用Python的标准库中的`open()`函数打开.log文件,然后使用正则表达式或其他方法来提取数据,最后使用Python的第三方库如`pandas`将数据存储到Excel表格中。以下是一个简单的代码示例:
```python
import re
import pandas as pd
# 打开.log文件
with open('example.log', 'r') as f:
log_data = f.read()
# 使用正则表达式提取数据
pattern = r'\[(\d+)\] (\w+): (.+)'
matches = re.findall(pattern, log_data)
# 将数据存储到DataFrame中
df = pd.DataFrame(matches, columns=['timestamp', 'level', 'message'])
# 将DataFrame存储到Excel表格中
df.to_excel('example.xlsx', index=False)
```
这段代码会将.log文件中的数据提取出来,存储到一个名为`example.xlsx`的Excel表格中。你可以根据自己的需要修改代码中的正则表达式和文件名。
阅读全文
相关推荐
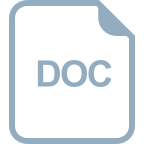
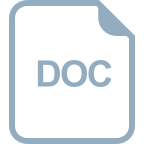

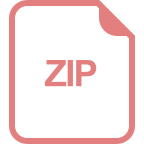
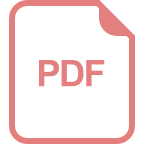
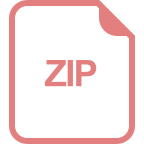
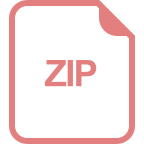
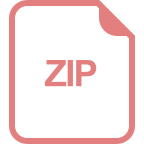
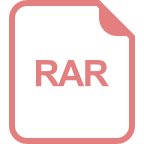
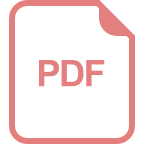
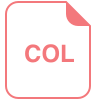
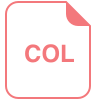
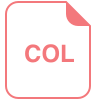
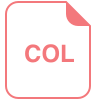


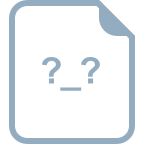
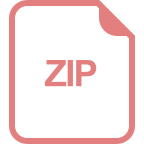