写一个安卓代码,要求如下:在MsgListActivity中点击任意消息条目跳转到ChatActivity,并将好友姓名传递过来。显示“你正在与”+好友姓名+“聊天\n你发送的消息:”。点击“发送”按钮,将在EditText中输入内容显示在上方TextView上。按下返回键,将最后一条消息内容返回至MsgListActivity,并更新好友姓名对应消息条目中的消息文本内容。
时间: 2024-03-24 15:39:42 浏览: 104
好的,以下是安卓代码的实现:
MsgListActivity.java
```java
public class MsgListActivity extends AppCompatActivity {
private TextView tvMsg;
private String friendName;
private String lastMsg;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_msg_list);
tvMsg = findViewById(R.id.tv_msg);
// 点击任意消息条目跳转到ChatActivity
tvMsg.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(MsgListActivity.this, ChatActivity.class);
intent.putExtra("friendName", friendName);
startActivityForResult(intent, 1);
}
});
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == RESULT_OK && requestCode == 1) {
// 获取返回的最后一条消息内容并更新UI
lastMsg = data.getStringExtra("lastMsg");
tvMsg.setText(friendName + ": " + lastMsg);
}
}
// 更新好友姓名对应消息条目中的消息文本内容
public void updateMsg(String name, String msg) {
friendName = name;
lastMsg = msg;
tvMsg.setText(friendName + ": " + lastMsg);
}
}
```
ChatActivity.java
```java
public class ChatActivity extends AppCompatActivity {
private EditText etSendMsg;
private TextView tvChat;
private String friendName;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_chat);
etSendMsg = findViewById(R.id.et_send_msg);
tvChat = findViewById(R.id.tv_chat);
// 获取好友姓名
friendName = getIntent().getStringExtra("friendName");
setTitle("正在与" + friendName + "聊天");
// 显示“你发送的消息:”
etSendMsg.setOnEditorActionListener(new TextView.OnEditorActionListener() {
@Override
public boolean onEditorAction(TextView v, int actionId, KeyEvent event) {
if (actionId == EditorInfo.IME_ACTION_SEND) {
String msg = v.getText().toString();
tvChat.setText("你发送的消息:" + msg);
v.setText("");
// 返回最后一条消息内容给MsgListActivity
Intent intent = new Intent();
intent.putExtra("lastMsg", msg);
setResult(RESULT_OK, intent);
return true;
}
return false;
}
});
}
@Override
public void onBackPressed() {
// 返回最后一条消息内容给MsgListActivity
String msg = tvChat.getText().toString().replace("你发送的消息:", "");
Intent intent = new Intent();
intent.putExtra("lastMsg", msg);
setResult(RESULT_OK, intent);
super.onBackPressed();
}
}
```
activity_msg_list.xml
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/ll_msg"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center_vertical"
android:orientation="vertical"
android:padding="16dp">
<TextView
android:id="@+id/tv_msg"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="好友姓名: 最后一条消息"
android:textSize="18sp" />
</LinearLayout>
```
activity_chat.xml
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/ll_chat"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="16dp">
<TextView
android:id="@+id/tv_chat"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="18sp" />
<EditText
android:id="@+id/et_send_msg"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="输入消息"
android:imeOptions="actionSend"
android:inputType="text" />
</LinearLayout>
```
阅读全文
相关推荐
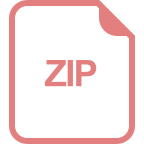
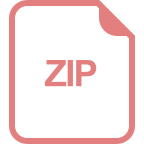
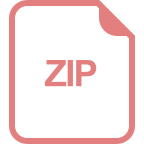

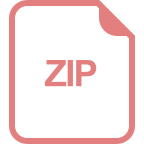
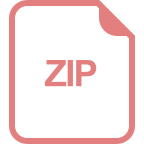
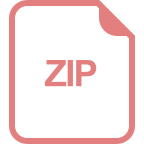
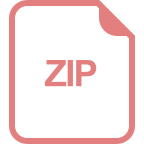
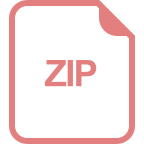
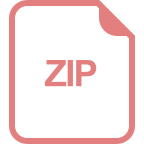
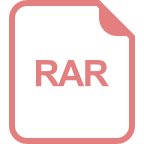
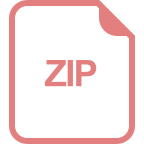
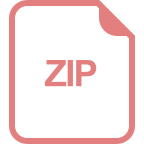
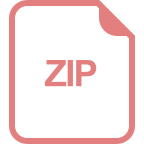
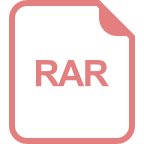
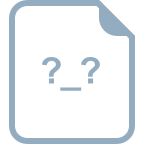
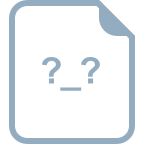
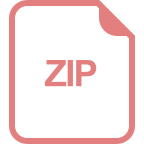
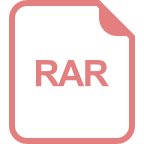
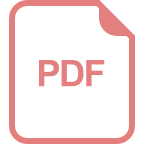