请用python编写在tensorflow框架下注意力机制的代码
时间: 2023-09-17 08:05:06 浏览: 71
### 回答1:
下面是一个使用TensorFlow实现注意力机制的示例代码:
```
import tensorflow as tf
class Attention(tf.keras.layers.Layer):
def __init__(self, units):
super(Attention, self).__init__()
self.W1 = tf.keras.layers.Dense(units)
self.W2 = tf.keras.layers.Dense(units)
self.V = tf.keras.layers.Dense(1)
def call(self, query, values):
# 对query和values进行线性变换
hidden_with_time_axis = tf.expand_dims(query, 1)
score = self.V(tf.nn.tanh(self.W1(values) + self.W2(hidden_with_time_axis)))
# 计算attention权重
attention_weights = tf.nn.softmax(score, axis=1)
# 计算context vector
context_vector = attention_weights * values
context_vector = tf.reduce_sum(context_vector, axis=1)
return context_vector, attention_weights
```
这是一个基本的注意力机制实现,您可以根据自己的需求进行扩展。
### 回答2:
以下是一个使用TensorFlow框架编写注意力机制的简单示例代码:
```python
import tensorflow as tf
class Attention(tf.keras.layers.Layer):
def __init__(self, units):
super(Attention, self).__init__()
self.W1 = tf.keras.layers.Dense(units)
self.W2 = tf.keras.layers.Dense(units)
self.V = tf.keras.layers.Dense(1)
def call(self, query, values):
query_with_time_axis = tf.expand_dims(query, 1)
score = tf.nn.tanh(self.W1(query_with_time_axis) + self.W2(values))
attention_weights = tf.nn.softmax(self.V(score), axis=1)
context_vector = attention_weights * values
context_vector = tf.reduce_sum(context_vector, axis=1)
return context_vector, attention_weights
class MyModel(tf.keras.Model):
def __init__(self, units, vocab_size):
super(MyModel, self).__init__()
self.embedding = tf.keras.layers.Embedding(vocab_size, units)
self.gru = tf.keras.layers.GRU(units, return_sequences=True)
self.attention = Attention(units)
self.fc = tf.keras.layers.Dense(vocab_size)
def call(self, inputs):
x = self.embedding(inputs)
hidden = self.gru(x)
context_vector, attention_weights = self.attention(hidden, x)
output = self.fc(context_vector)
return output, attention_weights
# 测试代码
units = 32
vocab_size = 10000
model = MyModel(units, vocab_size)
input_seq = tf.random.uniform((16, 10))
output, attention_weights = model(input_seq)
print(output.shape)
print(attention_weights.shape)
```
在这个示例代码中,我们首先定义了一个自定义的Attention层,其中使用了全连接层(Dense)和softmax函数计算注意力权重。接下来,我们定义了一个简单的模型MyModel,其中包括嵌入层(Embedding)、GRU层、Attention层和全连接层。最后,我们使用随机生成的输入序列进行模型的前向传播,并输出输出张量的形状和注意力权重的形状。
请注意,这只是一个简单的注意力机制示例代码,您可以根据自己的需求进行调整和扩展。
相关推荐
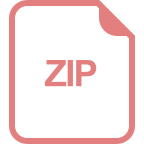
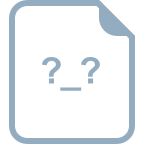












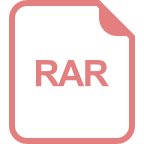
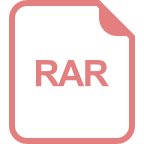