Microsoft.AspNetCore.Mvc.ControllerBase.File
时间: 2024-04-04 08:32:13 浏览: 110
Microsoft.AspNetCore.Mvc.ControllerBase.File 方法是一个控制器方法,用于返回指定文件的文件流。它接受文件路径、文件类型和文件下载的名称等参数,并返回一个 FileContentResult 对象,该对象包含文件的内容和相应的响应头信息,以便浏览器可以正确处理文件下载。该方法通常用于生成动态生成的文件,例如 PDF 报告、Excel 表格等。
相关问题
c#在.net框架下写一个下载大文件的api接口 不使用using Microsoft.AspNetCore.Mvc;
在.NET框架下,特别是没有`Microsoft.AspNetCore.Mvc`的情况下,我们可以创建一个简单的API接口来处理文件下载。这里我们假设你正在使用基础的ASP.NET Core Web API。首先,你需要设置HTTP响应头来表示这是一个下载操作,并提供一个POST请求来接收文件地址。
```csharp
using System;
using System.IO;
using System.Net.Http;
using System.Threading.Tasks;
namespace YourNamespace.Controllers // 请替换为你项目的实际命名空间
{
public class DownloadController : ControllerBase // 控制器继承自ApiController
{
[HttpPost("download")]
public async Task<IActionResult> DownloadFile([FromBody] string filePath)
{
if (string.IsNullOrEmpty(filePath))
{
return BadRequest("Please provide a valid file path.");
}
try
{
var httpClient = new HttpClient();
HttpResponseMessage response = await httpClient.GetAsync(filePath);
if (!response.IsSuccessStatusCode)
{
return StatusCode(response.StatusCode, "Failed to download file.");
}
var fileName = Path.GetFileName(filePath);
var memoryStream = new MemoryStream();
await response.Content.CopyToAsync(memoryStream);
// 设置响应头,告诉浏览器这是下载
Response.Headers.Add("Content-Disposition", $"attachment; filename={fileName}");
Response.ContentType = response.Content.Headers.ContentType.MediaType;
return File(memoryStream.ToArray(), Response.ContentType);
}
catch (Exception ex)
{
return StatusCode(500, "An error occurred while downloading the file: " + ex.Message);
}
}
}
}
```
在这个示例中,用户通过发送一个包含文件路径的POST请求到`/download`端点,API会尝试从该URL获取文件内容并将其作为附件返回给客户端。如果请求失败,将返回错误状态码和消息。
using System; using System.IO; using System.Linq; using Microsoft.AspNetCore.Http; using Microsoft.AspNetCore.Mvc; namespace FileUpload.Controllers { [ApiController] public class UploadController : ControllerBase { private const int ChunkSize = 1024 * 1024 * 1; // 每个分片的大小,这里设为1MB private const string UploadPath = "uploads"; // 文件上传目录 private static string _filePath; // 完整的文件路径 [HttpPost("/upload/start")] public ActionResult StartUpload(IFormFile file) { if (file == null || file.Length <= 0) { return BadRequest("请选择要上传的文件"); } // 生成文件名 string fileName = file.FileName; string fileExt = Path.GetExtension(fileName); string newFileName = Guid.NewGuid().ToString("N") + fileExt; // 生成文件存储目录 string dirPath = Path.Combine(Directory.GetCurrentDirectory(), UploadPath); if (!Directory.Exists(dirPath)) { Directory.CreateDirectory(dirPath); } // 生成文件路径 _filePath = Path.Combine(dirPath, newFileName); // 返回上传开始的响应 return Ok(new { FileName = newFileName, ChunkSize, }); } [HttpPost("/upload/append")] public ActionResult AppendUpload(string fileName, int chunkIndex, int chunks, IFormFile chunk) { byte[] buffer = new byte[ChunkSize]; int bytesRead = 0; int start = chunkIndex * ChunkSize; // 分片开始位置 int end = Math.Min(start + ChunkSize, (int)chunk.Length); // 分片结束位置 using (Stream stream = chunk.OpenReadStream()) { using (FileStream fileStream = new FileStream(_filePath, FileMode.OpenOrCreate, FileAccess.Write, FileShare.Write)) { fileStream.Position = start; while ((bytesRead = stream.Read(buffer, 0, Math.Min(buffer.Length, end - start))) > 0) { fileStream.Write(buffer, 0, bytesRead); start += bytesRead; } fileStream.Flush(true); } } // 检查是否所有分片都上传成功 int[] uploadedChunks = Directory.GetFiles(Path.GetDirectoryName(_filePath), $"{Path.GetFileName(_filePath)}_*").Select(filepath => int.Parse(filepath.Split('_')[1])).ToArray(); if (uploadedChunks.Length == chunks) { // 合并分片 using (FileStream fileStream = new FileStream(_filePath, FileMode.OpenOrCreate, FileAccess.Write, FileShare.Write)) { foreach (int index in uploadedChunks.OrderBy(i => i)) { string chunkPath = $"{_filePath}_{index}"; using (FileStream chunkStream = new FileStream(chunkPath, FileMode.Open, FileAccess.Read, FileShare.Read)) { chunkStream.CopyTo(fileStream); } System.IO.File.Delete(chunkPath); // 删除已合并的分片 } fileStream.Flush(true); } } return Ok(); } } }
这段代码是一个用于文件上传的 ASP.NET Core 控制器,包含两个 POST 方法:StartUpload 和 AppendUpload。StartUpload 方法接收一个 IFormFile 对象,用于启动文件上传并返回文件名和每个分片的大小。AppendUpload 方法接收文件名、当前分片索引、总分片数和当前分片数据,将当前分片写入文件并检查是否所有分片都上传成功,最后返回 Ok 结果。
阅读全文
相关推荐
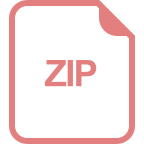
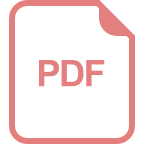
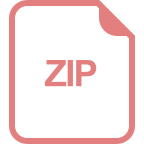













