vue3 typescript 获取大幅图片的缩略图并显示
时间: 2023-08-08 09:05:10 浏览: 74
你可以使用 HTML5 的 Canvas 元素来获取大幅图片的缩略图并显示。具体步骤如下:
1. 创建一个 HTML5 的 Canvas 元素。
2. 使用 JavaScript 的 Image 对象加载大幅图片。
3. 在 Image 对象的 onload 事件中,通过 Canvas 的 drawImage 方法将大幅图片绘制到 Canvas 上,并设置目标尺寸。
4. 使用 Canvas 的 toDataURL 方法将 Canvas 生成的缩略图转换为 Base64 编码的字符串。
5. 将 Base64 编码的字符串设置为 img 元素的 src 属性,即可显示缩略图。
下面是一个简单的实现示例:
```typescript
import { ref } from 'vue';
export default {
setup() {
const thumbnail = ref('');
const createThumbnail = (file: File) => {
const canvas = document.createElement('canvas');
const ctx = canvas.getContext('2d');
const img = new Image();
img.src = URL.createObjectURL(file);
img.onload = () => {
const { width, height } = img;
const targetWidth = 300;
const targetHeight = (height * targetWidth) / width;
canvas.width = targetWidth;
canvas.height = targetHeight;
ctx.drawImage(img, 0, 0, targetWidth, targetHeight);
thumbnail.value = canvas.toDataURL();
};
};
const handleChange = (event: Event) => {
const target = event.target as HTMLInputElement;
const file = target.files && target.files[0];
if (file) {
createThumbnail(file);
}
};
return {
thumbnail,
handleChange,
};
},
};
```
在模板中,可以使用 v-if 或 v-show 根据 thumbnail 的值来显示或隐藏 img 元素。
```html
<template>
<div>
<input type="file" @change="handleChange" />
<img v-if="thumbnail" :src="thumbnail" />
</div>
</template>
```
相关推荐
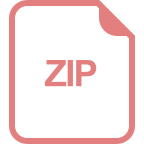
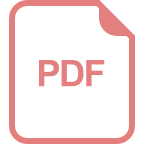














