c# List<string> 随机选取50个元素
时间: 2023-12-03 16:03:11 浏览: 30
可以使用C#中的Random类和List的Count属性来实现。具体代码如下:
```csharp
List<string> list = new List<string>(); // 假设这里已经有了字符串列表
Random rand = new Random();
List<string> randomList = new List<string>();
int count = 50; // 随机选取的元素个数
while (randomList.Count < count && list.Count > 0)
{
int index = rand.Next(list.Count);
randomList.Add(list[index]);
list.RemoveAt(index);
}
// randomList 中就是随机选取的50个元素
```
这段代码先创建了一个空的字符串列表`randomList`,然后使用`Random`类生成随机数,从原列表`list`中随机取出一个元素,添加到`randomList`中,并从`list`中删除该元素。重复此操作直到`randomList`中有50个元素或者`list`中没有元素为止。最后,`randomList`就是随机选取的50个元素。
相关问题
C#list<string>
以下是使用C#的List<string>泛型类的示例:
```csharp
List<string> mList = new List<string>();
mList.Add("apple");
mList.Add("banana");
mList.Add("orange");
// 删除长度大于3的字符串
mList.RemoveAll(name => name.Length > 3);
// 遍历列表并输出元素
foreach (string s in mList)
{
Console.WriteLine("element in mList: " + s);
}
```
输出结果:
```
element in mList: apple
```
这个示例演示了如何使用List<string>泛型类创建一个字符串列表,并使用RemoveAll方法删除长度大于3的字符串。然后,使用foreach循环遍历列表并输出每个元素。
C#List<List<string>>转成二维数组
将`List<List<string>>`转换为二维数组可以使用循环遍历,将内部的List<string>`转换为一维数组,再将这些一维数组添加到二维数组中。以下是一个示例代码:
```csharp
List<List<string>> data = new List<List<string>>
{
new List<string> {"A1", "B1", "C1"},
new List<string> {"A2", "B2", "C2"},
new List<string> {"A3", "B3", "C3"},
};
// 获取二维数组的行数和列数
int rowCount = data.Count;
int columnCount = data[0].Count;
// 初始化二维数组
string[,] array = new string[rowCount, columnCount];
// 遍历List,将每个内部的List<string>转换为一维数组,再添加到二维数组中
for (int i = 0; i < rowCount; i++)
{
for (int j = 0; j < columnCount; j++)
{
array[i, j] = data[i][j];
}
}
```
在这个例子中,我们首先获取了`List<List<string>>`的行数和列数,并创建了一个相同大小的二维数组`array`。然后,我们使用双重循环遍历`List<List<string>>`,将每个内部的`List<string>`转换为一维数组,再将其添加到二维数组中。最终,我们得到了一个二维数组`array`,其中包含了`List<List<string>>`中的所有数据。
相关推荐
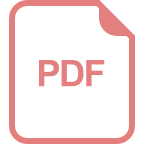












