python image stitching
时间: 2024-05-27 19:12:40 浏览: 29
Python image stitching is the process of combining multiple images with overlapping areas to create a panoramic or panoramic-like image. This is often used in landscape photography and can be achieved using various image processing techniques.
相关问题
Python realizes panoramic stitching using optical flow tracing principle
Panoramic stitching is the process of combining multiple images to create a single panoramic image. Optical flow tracing is a technique used to track the movement of pixels between consecutive frames in a video or between multiple images.
Here's an example of how you can implement panoramic stitching using the optical flow tracing principle in Python:
1. Start by importing the necessary libraries, such as OpenCV, Numpy, and Matplotlib.
```
import cv2
import numpy as np
import matplotlib.pyplot as plt
```
2. Load the images that you want to stitch into a list.
```
images = [cv2.imread('image1.jpg'), cv2.imread('image2.jpg'), ... ]
```
3. Use the Lucas-Kanade optical flow method to track the movement of pixels between the images.
```
gray_images = [cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) for img in images]
prev_gray = gray_images[0]
flow = None
for i in range(1, len(gray_images)):
curr_gray = gray_images[i]
flow = cv2.calcOpticalFlowFarneback(prev_gray, curr_gray, flow, 0.5, 3, 15, 3, 5, 1.2, 0)
prev_gray = curr_gray
```
4. Use the optical flow information to warp the images and align them.
```
warped_images = []
for i in range(len(images)):
H = np.eye(3)
for j in range(i):
H = np.dot(H, cv2.estimateRigidTransform(gray_images[j], gray_images[i], False))
warped = cv2.warpPerspective(images[i], H, (images[i].shape[1] + int(H[0,2]), images[i].shape[0] + int(H[1,2])))
warped_images.append(warped)
```
5. Finally, use the aligned images to create a single panoramic image.
```
panorama = warped_images[0]
for i in range(1, len(warped_images)):
panorama = cv2.addWeighted(panorama, 1, warped_images[i], 1, 0)
```
This is just a basic example, and you may need to adjust the parameters or use a different approach depending on your specific use case. But I hope it gives you an idea of how to implement panoramic stitching using the optical flow tracing principle in Python.
SSDA图像拼接python
SSDA图像拼接是一种通过仿射变换和扭曲操作将多个图像拼接在一起的技术。你可以使用Python中的OpenCV库和SciPy工具包来实现SSDA图像拼接。
首先,你需要导入所需的库并加载待拼接的图像。然后,你可以使用仿射变换和扭曲操作将图像进行拼接。具体步骤如下:
1. 导入必要的库:
```python
import cv2
from scipy import ndimage
from PIL import Image
```
2. 加载图像:
```python
im1 = cv2.imread('image1.jpg')
im2 = cv2.imread('image2.jpg')
```
3. 定义扭曲矩阵:
```python
H = np.array([[1.4, 0.05, -100], [0.05, 1.5, -100], [0, 0, 1]])
```
4. 进行仿射变换和扭曲操作:
```python
im2_warped = cv2.warpPerspective(im2, H, (im1.shape[1]+im2.shape[1], im1.shape[0]))
im2_warped[0:im1.shape[0], 0:im1.shape[1]] = im1
```
5. 显示拼接结果:
```python
cv2.imshow('SSDA Image Stitching', im2_warped)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
请注意,以上代码仅为示例,你需要根据你的具体需求进行适当的调整。
相关推荐
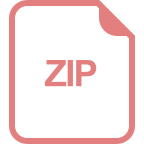
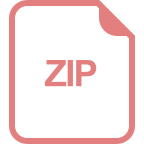
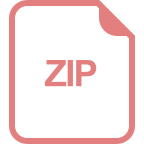












