分裂聚类实现鸢尾花数据集聚类并可视化
时间: 2023-08-20 10:05:50 浏览: 106
分裂聚类(Divisive clustering)是一种自上而下的聚类方法,它首先将所有数据点视为一个簇,然后递归地将每个簇分成更小的簇,直到满足某个停止准则为止。这个停止准则可以是簇的数量达到预设的数量,或者簇内距离的方差小于某个阈值等。
下面演示如何使用分裂聚类算法实现鸢尾花数据集聚类并可视化。
首先,我们需要导入必要的库和加载鸢尾花数据集。
```python
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from sklearn.datasets import load_iris
iris = load_iris()
X = iris.data
y = iris.target
```
接下来,我们定义一个分裂聚类函数 `divisive_clustering`,它接收数据集 `X` 和停止准则 `stop_criterion` 作为参数,返回所有簇的标签。
```python
def divisive_clustering(X, stop_criterion):
labels = np.zeros(X.shape[0])
cluster_count = 1
recursive_divisive_clustering(X, labels, 0, stop_criterion, cluster_count)
return labels
def recursive_divisive_clustering(X, labels, current_cluster, stop_criterion, cluster_count):
cluster_indices = np.where(labels == current_cluster)[0]
if cluster_indices.size == 0:
return
if stop_criterion(X[cluster_indices]):
return
left_cluster_indices, right_cluster_indices = split_cluster(X[cluster_indices])
if left_cluster_indices.size > 0:
labels[cluster_indices[left_cluster_indices]] = cluster_count
cluster_count += 1
recursive_divisive_clustering(X, labels, current_cluster + 1, stop_criterion, cluster_count)
if right_cluster_indices.size > 0:
labels[cluster_indices[right_cluster_indices]] = cluster_count
cluster_count += 1
recursive_divisive_clustering(X, labels, current_cluster + 1, stop_criterion, cluster_count)
def split_cluster(X):
# Find the feature with the largest variance
variances = np.var(X, axis=0)
split_feature = np.argmax(variances)
# Split the cluster by the median of the selected feature
median = np.median(X[:, split_feature])
left_cluster_indices = np.where(X[:, split_feature] < median)[0]
right_cluster_indices = np.where(X[:, split_feature] >= median)[0]
return left_cluster_indices, right_cluster_indices
```
在上述代码中,我们使用递归函数 `recursive_divisive_clustering` 实现分裂聚类。在每次递归中,我们使用 `split_cluster` 函数将当前簇分成左右两个子簇,并将子簇中的数据点分别标记为新的簇标签。如果子簇数量大于1,则继续递归分裂子簇,直到满足停止准则为止。
停止准则 `stop_criterion` 是一个函数,它接收一个簇的数据点作为参数,并返回一个布尔值,表示是否满足停止条件。
下面我们定义一个停止准则函数 `variance_stop_criterion`,它判断簇内距离的方差是否小于某个阈值 `threshold`。
```python
def variance_stop_criterion(X, threshold=1.0):
return np.var(X) < threshold
```
最后,我们调用分裂聚类函数 `divisive_clustering`,并将聚类结果可视化。
```python
labels = divisive_clustering(X, variance_stop_criterion)
plt.scatter(X[:, 0], X[:, 1], c=labels)
plt.xlabel('sepal length (cm)')
plt.ylabel('sepal width (cm)')
plt.show()
```
运行上述代码,我们可以看到鸢尾花数据集被分成了多个簇,并且每个簇被用不同的颜色标记。

需要注意的是,在实际应用中,分裂聚类算法的效率不如层次聚类算法和K均值聚类算法。因此,分裂聚类算法通常只用于数据量较小的情况下。
阅读全文
相关推荐
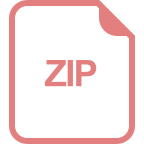










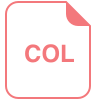
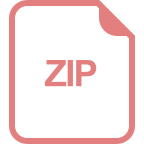
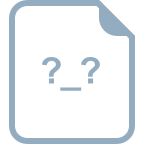