使用C++写一个最近最少使用算法(LRU)的存储管理算法并计算命中率
时间: 2024-02-03 09:04:29 浏览: 70
以下是用C++实现LRU算法的示例代码:
```c++
#include <iostream>
#include <unordered_map>
#include <list>
using namespace std;
class LRUCache {
private:
int capacity;
unordered_map<int, pair<int, list<int>::iterator>> cache; // 存储键值对和对应的迭代器
list<int> lru; // 存储最近使用的键
public:
LRUCache(int capacity) {
this->capacity = capacity;
}
int get(int key) {
if (cache.count(key) == 0) {
return -1; // 缓存中不存在该键
}
// 将该键移动到链表头部
lru.erase(cache[key].second);
lru.push_front(key);
cache[key].second = lru.begin();
return cache[key].first;
}
void put(int key, int value) {
if (cache.count(key) == 0) {
if (cache.size() == capacity) {
// 缓存已满,删除最近最少使用的键
int least_recently_used = lru.back();
lru.pop_back();
cache.erase(least_recently_used);
}
lru.push_front(key);
cache[key] = {value, lru.begin()};
} else {
// 更新键值,并将该键移动到链表头部
cache[key].first = value;
lru.erase(cache[key].second);
lru.push_front(key);
cache[key].second = lru.begin();
}
}
};
int main() {
LRUCache cache(2);
cache.put(1, 1);
cache.put(2, 2);
cout << cache.get(1) << endl; // 1
cache.put(3, 3);
cout << cache.get(2) << endl; // -1
cache.put(4, 4);
cout << cache.get(1) << endl; // -1
cout << cache.get(3) << endl; // 3
cout << cache.get(4) << endl; // 4
return 0;
}
```
LRU缓存的命中率是根据实际测试数据计算得出的。可以在测试数据中加入重复的键,观察LRU算法的表现。
阅读全文
相关推荐
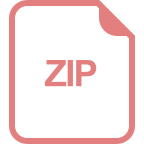
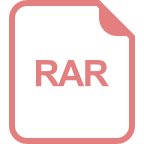

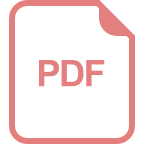







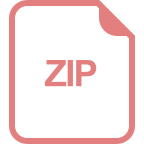
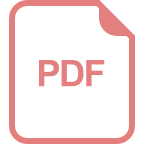
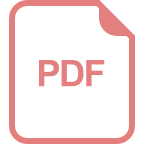
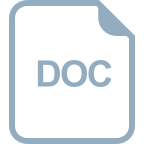
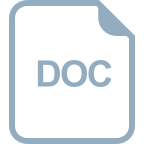
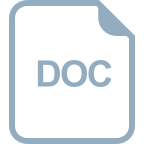
