写一个java记事本中‘字数显示’的代码
时间: 2024-03-05 08:49:37 浏览: 76
下面是一个简单的Java记事本中实现“字数显示”的代码:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Notepad extends JFrame implements ActionListener {
private JTextArea textArea;
private JLabel statusLabel;
public Notepad() {
super("记事本");
// 创建菜单栏
JMenuBar menuBar = new JMenuBar();
setJMenuBar(menuBar);
// 创建文件菜单
JMenu fileMenu = new JMenu("文件");
menuBar.add(fileMenu);
// 创建打开菜单项
JMenuItem openMenuItem = new JMenuItem("打开");
openMenuItem.addActionListener(this);
fileMenu.add(openMenuItem);
// 创建保存菜单项
JMenuItem saveMenuItem = new JMenuItem("保存");
saveMenuItem.addActionListener(this);
fileMenu.add(saveMenuItem);
// 创建编辑区域
textArea = new JTextArea();
JScrollPane scrollPane = new JScrollPane(textArea);
add(scrollPane, BorderLayout.CENTER);
// 创建状态栏
statusLabel = new JLabel("字数: 0");
add(statusLabel, BorderLayout.SOUTH);
// 设置窗口大小和关闭操作
setSize(500, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public void actionPerformed(ActionEvent e) {
// 处理打开和保存菜单项的事件
// ...
// 更新字数显示
String text = textArea.getText();
int count = text.length();
statusLabel.setText("字数: " + count);
}
public static void main(String[] args) {
Notepad notepad = new Notepad();
notepad.setVisible(true);
}
}
```
在上面的代码中,我们创建了一个JLabel组件来显示字数,并在actionPerformed方法中更新它的文本。每当用户打开、保存或编辑文本时,我们都会计算文本的长度,并将其显示在状态栏中。
阅读全文
相关推荐
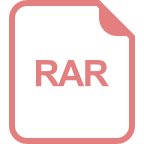
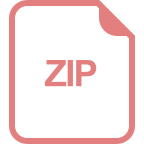
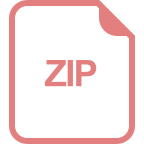
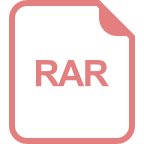
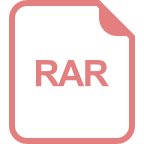
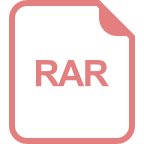
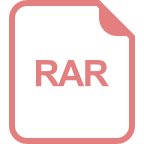
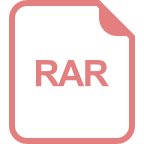
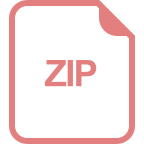
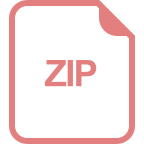
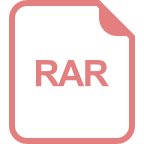
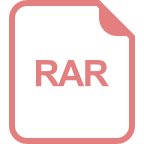
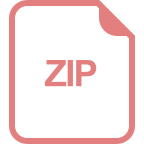
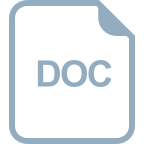
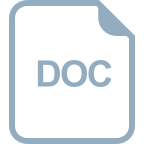