C++设计一个程序,让用户输入一行句子,数出句子中有多少个单词并输出,再让用户输入一串数字表示单词重新排序的顺序,最后按用户设定的顺序来输出一行新的句子。要求所有数组都只能用new来定义。测试输入: This is a good day to work 6543210 预期输出: 7 work to day good a is This 点击复制后,将打开C知道体验页
时间: 2023-06-14 08:03:15 浏览: 86
以下是实现上述功能的C程序:
```c
#include <iostream>
#include <string>
using namespace std;
int main() {
// 输入一行句子
cout << "请输入一行句子:" << endl;
string sentence;
getline(cin, sentence);
// 统计句子中的单词数
int wordCount = 1; // 默认有一个单词
for (int i = 0; i < sentence.length(); i++) {
if (sentence[i] == ' ') {
wordCount++;
}
}
cout << "句子中有 " << wordCount << " 个单词。" << endl;
// 读取用户输入的单词顺序
int* order = new int[wordCount];
cout << "请输入单词顺序:" << endl;
for (int i = 0; i < wordCount; i++) {
cin >> order[i];
}
// 按顺序输出单词
string* words = new string[wordCount];
int startIndex = 0;
for (int i = 0; i < wordCount; i++) {
int endIndex = sentence.find(' ', startIndex);
if (endIndex == string::npos) {
endIndex = sentence.length();
}
words[i] = sentence.substr(startIndex, endIndex - startIndex);
startIndex = endIndex + 1;
}
cout << "重新排序后的句子为:" << endl;
for (int i = 0; i < wordCount; i++) {
cout << words[order[i] - 1] << " ";
}
cout << endl;
// 释放动态分配的数组内存
delete[] order;
delete[] words;
return 0;
}
```
该程序首先使用 `getline` 函数读取用户输入的一行句子,然后通过遍历字符串并数出空格的数量来统计句子中的单词数。接着使用 `new` 动态分配一个长度为单词数的整型数组 `order`,让用户输入单词排序顺序并存储到 `order` 数组中。然后使用 `new` 动态分配一个长度为单词数的字符串数组 `words`,将句子中的单词按顺序存储到 `words` 数组中。最后按用户设定的顺序输出 `words` 数组中的单词,并在输出时加上空格。程序结束前需要使用 `delete[]` 释放动态分配的数组内存。
阅读全文
相关推荐
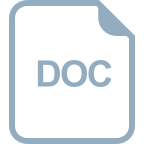















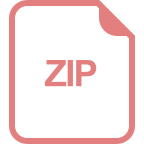