用C语言制作一款贪吃蛇
时间: 2023-06-13 13:05:08 浏览: 92
好的,下面是一份简单的贪吃蛇游戏的C代码,仅供参考:
```c
#include<stdio.h>
#include<stdlib.h>
#include<conio.h>
#include<windows.h>
#define WIDTH 60 // 地图宽度
#define HEIGHT 20 // 地图高度
int score = 0; // 分数
int speed = 200; // 蛇移动速度,单位ms
int direction = 1; // 蛇移动方向,1:向右,2:向下,3:向左,4:向上
struct Node // 链表结构体,用于存储蛇身体各节点的坐标
{
int x;
int y;
struct Node *next;
};
struct Node *head, *tail; // 头节点和尾节点
int map[HEIGHT][WIDTH]; // 地图数组,0:空白,1:蛇身体,2:食物
void gotoxy(int x, int y) // 光标移动函数
{
COORD pos = { x, y };
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), pos);
}
void init() // 初始化函数,用于生成初始蛇和食物
{
// 生成蛇头,坐标为(5, 5)
head = (struct Node*)malloc(sizeof(struct Node));
head->x = 5;
head->y = 5;
head->next = NULL;
map[5][5] = 1;
// 生成蛇身体,坐标为(4, 5)
struct Node *body = (struct Node*)malloc(sizeof(struct Node));
body->x = 4;
body->y = 5;
body->next = head;
head = body;
map[4][5] = 1;
// 生成蛇身体,坐标为(3, 5)
tail = (struct Node*)malloc(sizeof(struct Node));
tail->x = 3;
tail->y = 5;
tail->next = body;
body->next = tail;
map[3][5] = 1;
// 生成食物,随机坐标
int x, y;
do {
x = rand() % HEIGHT;
y = rand() % WIDTH;
} while (map[x][y] != 0);
map[x][y] = 2;
// 绘制初始界面
gotoxy(0, 0);
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
if (map[i][j] == 0) {
printf(" ");
}
else if (map[i][j] == 1) {
printf("*");
}
else if (map[i][j] == 2) {
printf("#");
}
}
printf("\n");
}
}
void addNode(int x, int y) // 添加新节点到蛇身体的头部
{
struct Node *node = (struct Node*)malloc(sizeof(struct Node));
node->x = x;
node->y = y;
node->next = head;
head = node;
}
void deleteNode() // 删除蛇尾节点
{
struct Node *p = tail;
tail = tail->next;
map[p->x][p->y] = 0;
free(p);
}
void move() // 蛇移动函数
{
int x = head->x;
int y = head->y;
// 计算蛇头移动后的坐标
if (direction == 1) {
y++;
}
else if (direction == 2) {
x++;
}
else if (direction == 3) {
y--;
}
else if (direction == 4) {
x--;
}
// 判断是否吃到食物
if (map[x][y] == 2) {
addNode(x, y);
score++;
// 生成新的食物,随机坐标
int tx, ty;
do {
tx = rand() % HEIGHT;
ty = rand() % WIDTH;
} while (map[tx][ty] != 0);
map[tx][ty] = 2;
}
else {
addNode(x, y);
deleteNode();
}
// 判断是否撞到墙或自己的身体
if (x < 0 || x >= HEIGHT || y < 0 || y >= WIDTH || map[x][y] == 1) {
printf("Game Over! Your score is %d.\n", score);
exit(0);
}
// 更新地图
map[x][y] = 1;
map[head->next->x][head->next->y] = 1;
// 绘制界面
gotoxy(0, 0);
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
if (map[i][j] == 0) {
printf(" ");
}
else if (map[i][j] == 1) {
printf("*");
}
else if (map[i][j] == 2) {
printf("#");
}
}
printf("\n");
}
}
int main()
{
init(); // 初始化
while (1) {
Sleep(speed); // 控制蛇移动速度
if (_kbhit()) { // 判断是否有按键输入
char c = _getch();
if (c == 'a' && direction != 1) {
direction = 3;
}
else if (c == 's' && direction != 4) {
direction = 2;
}
else if (c == 'd' && direction != 3) {
direction = 1;
}
else if (c == 'w' && direction != 2) {
direction = 4;
}
}
move(); // 蛇移动
}
return 0;
}
```
这份代码使用了链表来存储蛇身体各节点的坐标,以便于添加和删除节点。在初始化函数中,生成了初始的蛇身体和食物,并绘制了初始界面。在蛇移动函数中,实现了蛇的移动、吃食物、判断是否撞到墙或自己的身体,更新地图,并绘制界面。在主函数中,使用了`_kbhit()`和`_getch()`函数来判断是否有按键输入,并根据不同的按键更新蛇移动方向。
阅读全文
相关推荐

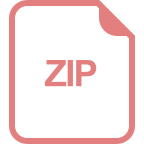
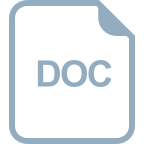
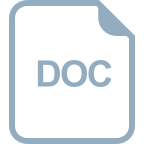
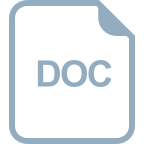
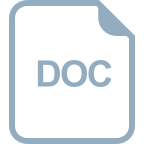
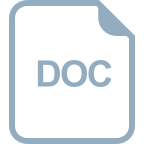

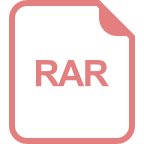
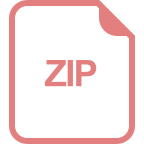
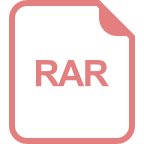
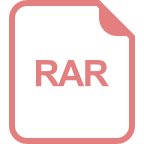
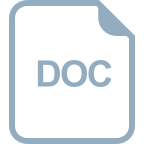
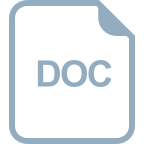