windows下c++使用libHaru库开发指定纸张和纸盒将pdf发送到打印机进行打印的程序
时间: 2024-05-14 07:14:37 浏览: 111
以下是使用libHaru库开发指定纸张和纸盒将pdf发送到打印机进行打印的程序的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <windows.h>
#include "hpdf.h"
#define WIDTH 595
#define HEIGHT 842
void error_handler(HPDF_STATUS error_no, HPDF_STATUS detail_no, void *user_data) {
printf("ERROR: error_no=%04X, detail_no=%u\n", (unsigned int)error_no, (unsigned int)detail_no);
exit(1);
}
int main()
{
HPDF_Doc pdfDoc = NULL;
HPDF_Page pdfPage = NULL;
HPDF_Font pdfFont = NULL;
HPDF_Image pdfImage = NULL;
HPDF_REAL pageWidth = 0;
HPDF_REAL pageHeight = 0;
HPDF_STATUS pdfStatus = HPDF_OK;
const char *pdfFileName = "test.pdf";
const char *fontName = "Helvetica-Bold";
const char *imageFileName = "test.jpg";
const char *printerName = "Microsoft Print to PDF";
int pageIndex = 0;
int imageWidth = 0;
int imageHeight = 0;
int printWidth = 0;
int printHeight = 0;
// 初始化libHaru库
pdfDoc = HPDF_New(error_handler, NULL);
if (!pdfDoc) {
printf("ERROR: Cannot create PDF document.\n");
return 1;
}
// 创建新页面
pdfPage = HPDF_AddPage(pdfDoc);
if (!pdfPage) {
printf("ERROR: Cannot create PDF page.\n");
HPDF_Free(pdfDoc);
return 1;
}
// 设置页面大小
HPDF_Page_SetSize(pdfPage, HPDF_PAGE_SIZE_A4, HPDF_PAGE_PORTRAIT);
// 设置字体
pdfFont = HPDF_GetFont(pdfDoc, fontName, NULL);
if (!pdfFont) {
printf("ERROR: Cannot get font.\n");
HPDF_Free(pdfDoc);
return 1;
}
// 插入文本
HPDF_Page_SetFontAndSize(pdfPage, pdfFont, 24);
HPDF_Page_BeginText(pdfPage);
HPDF_Page_MoveTextPos(pdfPage, 220, 750);
HPDF_Page_ShowText(pdfPage, "Hello World!");
HPDF_Page_EndText(pdfPage);
// 插入图像
pdfImage = HPDF_LoadJpegImageFromFile(pdfDoc, imageFileName);
if (!pdfImage) {
printf("ERROR: Cannot load image.\n");
HPDF_Free(pdfDoc);
return 1;
}
imageWidth = HPDF_Image_GetWidth(pdfImage);
imageHeight = HPDF_Image_GetHeight(pdfImage);
printWidth = WIDTH;
printHeight = (int)((float)printWidth / (float)imageWidth * (float)imageHeight);
HPDF_Page_DrawImage(pdfPage, pdfImage, 0, 0, printWidth, printHeight);
// 保存PDF文件
pdfStatus = HPDF_SaveToFile(pdfDoc, pdfFileName);
if (pdfStatus != HPDF_OK) {
printf("ERROR: Cannot save PDF file.\n");
HPDF_Free(pdfDoc);
return 1;
}
// 获取页面大小
pageWidth = HPDF_Page_GetWidth(pdfPage);
pageHeight = HPDF_Page_GetHeight(pdfPage);
// 打印PDF文件
pageIndex = 0;
pdfStatus = HPDF_PrintWithOption(pdfDoc, printerName, NULL, pageIndex, pageIndex, NULL);
if (pdfStatus != HPDF_OK) {
printf("ERROR: Cannot print PDF file.\n");
HPDF_Free(pdfDoc);
return 1;
}
// 释放资源
HPDF_Free(pdfDoc);
return 0;
}
```
在上面的示例代码中,我们首先使用HPDF_New()函数初始化libHaru库,并创建一个新的PDF文档。然后,我们使用HPDF_AddPage()函数创建一个新的页面,并使用HPDF_Page_SetSize()函数设置页面的大小。接下来,我们使用HPDF_GetFont()函数获取指定的字体,并使用HPDF_Page_SetFontAndSize()函数设置字体和字号。然后,我们使用HPDF_Page_BeginText()函数开始插入文本,使用HPDF_Page_MoveTextPos()函数设置文本的位置,使用HPDF_Page_ShowText()函数插入文本,最后使用HPDF_Page_EndText()函数结束插入文本。接下来,我们使用HPDF_LoadJpegImageFromFile()函数从文件中加载JPEG图像,并使用HPDF_Image_GetWidth()和HPDF_Image_GetHeight()函数获取图像的宽度和高度。然后,我们计算图像在打印机上的打印宽度和打印高度,并使用HPDF_Page_DrawImage()函数将图像插入到页面中。最后,我们使用HPDF_SaveToFile()函数将PDF文件保存到磁盘上,并使用HPDF_PrintWithOption()函数将PDF文件发送到指定的打印机进行打印。最后,我们使用HPDF_Free()函数释放资源。
需要注意的是,在上面的示例代码中,我们使用了Windows API中的Microsoft Print to PDF虚拟打印机来进行打印。如果你要使用实际的打印机进行打印,需要将printerName参数替换为实际的打印机名称。此外,如果你要打印的PDF文件较大,可能需要使用HPDF_Page_Continue()函数将内容分成多个页面进行打印。
相关推荐
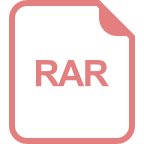
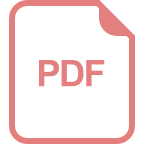
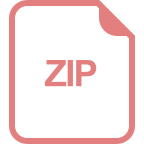














