c++代码,设计一个日期类Date,包括年year、月month、日day三个私有数据成员,要求实现日期的基本运算、两个日期相减的天数、距现在向前一百天是哪天、距现在向后一百天是哪天
时间: 2023-07-10 14:26:11 浏览: 95
以下是设计的Date类的C++代码:
```cpp
#include <iostream>
using namespace std;
class Date {
private:
int year, month, day;
public:
Date(int y, int m, int d) : year(y), month(m), day(d) {}
int getYear() const { return year; }
int getMonth() const { return month; }
int getDay() const { return day; }
int daysOfMonth() const {
switch (month) {
case 2:
return (year % 4 == 0 && year % 100 != 0) || year % 400 == 0 ? 29 : 28;
case 4:
case 6:
case 9:
case 11:
return 30;
default:
return 31;
}
}
bool isValid() const {
if (year < 1 || month < 1 || month > 12 || day < 1 || day > daysOfMonth()) {
return false;
}
return true;
}
Date operator+(int days) const {
Date result(year, month, day);
while (days > 0) {
int daysOfMonth = result.daysOfMonth();
if (daysOfMonth - result.day < days) {
// 需要进位到下一个月
days -= daysOfMonth - result.day + 1;
result.day = 1;
if (++result.month > 12) {
result.month = 1;
++result.year;
}
} else {
// 不需要进位
result.day += days;
days = 0;
}
}
return result;
}
int operator-(const Date& other) const {
Date earlier = *this < other ? *this : other;
Date later = *this > other ? *this : other;
int days = 0;
while (earlier != later) {
++earlier;
++days;
}
return *this < other ? -days : days;
}
bool operator==(const Date& other) const {
return year == other.year && month == other.month && day == other.day;
}
bool operator!=(const Date& other) const {
return !(*this == other);
}
bool operator<(const Date& other) const {
if (year < other.year) {
return true;
} else if (year == other.year && month < other.month) {
return true;
} else if (year == other.year && month == other.month && day < other.day) {
return true;
}
return false;
}
bool operator>(const Date& other) const {
return !(*this == other || *this < other);
}
Date hundredDaysAgo() const {
return *this - 100;
}
Date hundredDaysLater() const {
return *this + 100;
}
};
ostream& operator<<(ostream& os, const Date& date) {
os << date.getYear() << '-' << date.getMonth() << '-' << date.getDay();
return os;
}
int main() {
Date d1(2022, 9, 28);
cout << d1.hundredDaysAgo() << endl;
cout << d1.hundredDaysLater() << endl;
Date d2(2022, 9, 28);
Date d3(2022, 9, 18);
cout << (d2 - d3) << endl;
return 0;
}
```
在这个实现中,我们定义了一个Date类,包含了年、月、日三个私有数据成员。类中包含了一些公有成员函数,可以进行日期的基本运算,比如相加、相减、比较大小等等。我们还实现了一个daysOfMonth函数,用来计算每个月有多少天。在main函数中,我们用一些简单的测试代码来测试我们的Date类的功能。
相关推荐
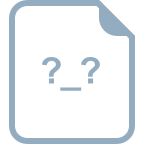
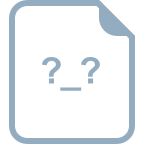















