springboot 从redis中取出json数据,请写出代码
时间: 2024-10-15 11:16:31 浏览: 26
在Spring Boot中,要从Redis获取JSON数据,你可以使用Spring Data Redis的`ValueOperations`接口。以下是一个简单的示例,展示如何使用JedisTemplate或 lettuce等Redis客户端操作工具:
**使用JedisTemplate:**
```java
import org.springframework.data.redis.core.StringRedisTemplate;
import org.springframework.data.redis.core.ValueOperations;
public class RedisService {
private final StringRedisTemplate stringRedisTemplate;
public RedisService(StringRedisTemplate stringRedisTemplate) {
this.stringRedisTemplate = stringRedisTemplate;
}
public Object getJsonFromRedis(String key) {
ValueOperations<String, String> operations = stringRedisTemplate.opsForValue();
String jsonContent = operations.get(key);
return jsonContent == null ? null : new ObjectMapper().readValue(jsonContent, YourObject.class); // 假设YourObject是你的JSON模型类
}
}
```
**使用lettuce:**
```java
import io.lettuce.core.api.StatefulRedisConnection;
import io.lettuce.core.api.async.RedisFuture;
import io.lettuce.core.json.JsonMapper;
public class RedisService {
private final LettuceConnectionFactory connectionFactory;
public RedisService(LettuceConnectionFactory connectionFactory) {
this.connectionFactory = connectionFactory;
}
public CompletableFuture<YourObject> getJsonFromRedis(String key) throws Exception {
StatefulRedisConnection<String, String> redis = connectionFactory.connect();
try {
String jsonContent = redis.get(key).get();
return CompletableFuture.completedFuture(JsonMapper.jsonObjectMapper().fromJson(jsonContent, YourObject.class));
} finally {
redis.close();
}
}
}
```
注意这里假设你已经有了一个`YourObject`类,这个类需要有对应的Jackson或Gson的反序列化方法来解析JSON字符串。
阅读全文
相关推荐
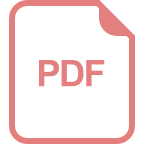
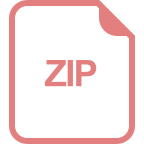
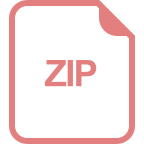
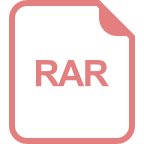
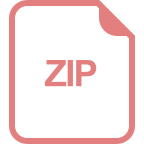
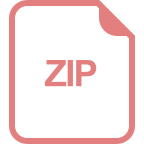
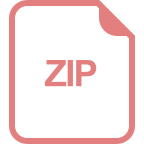
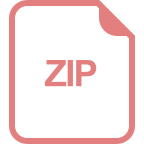
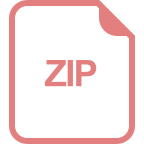
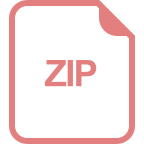
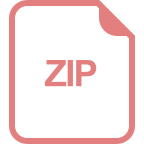
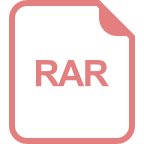
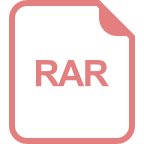
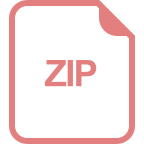
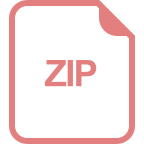
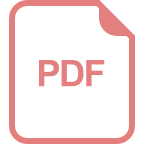