使用C++类 多态写一个四则运算,注意调用父类的数据成员并初始化
时间: 2024-05-06 20:16:34 浏览: 10
好的,以下是使用C++类多态写一个四则运算的示例代码:
```c++
#include <iostream>
using namespace std;
class Operation {
public:
virtual double getResult(double num1, double num2) {
return 0;
}
};
class AddOperation : public Operation {
public:
double getResult(double num1, double num2) {
return num1 + num2;
}
};
class SubOperation : public Operation {
public:
double getResult(double num1, double num2) {
return num1 - num2;
}
};
class MulOperation : public Operation {
public:
double getResult(double num1, double num2) {
return num1 * num2;
}
};
class DivOperation : public Operation {
public:
double getResult(double num1, double num2) {
if (num2 == 0) {
cout << "Error: Divided by zero!" << endl;
return 0;
}
return num1 / num2;
}
};
int main() {
double num1, num2;
char op;
cout << "Please enter the first number: ";
cin >> num1;
cout << "Please enter the operator (+, -, *, /): ";
cin >> op;
cout << "Please enter the second number: ";
cin >> num2;
Operation *operation;
switch (op) {
case '+':
operation = new AddOperation();
break;
case '-':
operation = new SubOperation();
break;
case '*':
operation = new MulOperation();
break;
case '/':
operation = new DivOperation();
break;
default:
cout << "Error: Invalid operator!" << endl;
return 0;
}
cout << num1 << " " << op << " " << num2 << " = " << operation->getResult(num1, num2) << endl;
delete operation;
return 0;
}
```
在这个示例代码中,我们定义了一个 `Operation` 类作为父类,并在其中定义了一个虚函数 `getResult()`,用于计算两个数字的运算结果。然后我们定义了四个子类 `AddOperation`、`SubOperation`、`MulOperation` 和 `DivOperation`,分别用于实现加法、减法、乘法和除法。
在 `main()` 函数中,我们通过用户输入的运算符来选择使用哪个子类,然后创建对应的对象并调用其 `getResult()` 方法计算结果,最后输出结果并释放对象内存。
需要注意的是,在父类中定义虚函数时,需要在函数前面加上 `virtual` 关键字,而在子类中重写该函数时,需要在函数前面加上 `override` 关键字。另外,在使用完动态分配的对象后,需要使用 `delete` 关键字手动释放对象内存,防止内存泄漏。
相关推荐
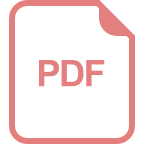
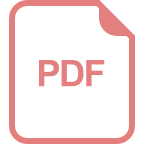
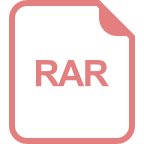














