怎么写一个为三个学生成绩排名的c语言代码
时间: 2024-09-28 17:14:20 浏览: 30
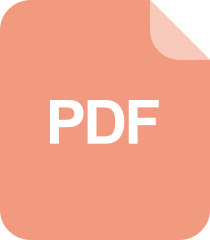
C语言编写学生成绩管理系统
在C语言中编写一个简单的程序来为三个学生的成绩排名,我们可以创建一个结构体表示学生,并包含姓名和成绩属性,然后定义一个函数来进行排序。下面是一个基本的例子:
```c
#include <stdio.h>
#include <string.h>
// 定义学生结构体
typedef struct {
char name[50];
float score;
} Student;
// 比较两个学生分数并返回排名
int compare(const void *a, const void *b) {
const Student *student1 = (const Student *)a;
const Student *student2 = (const Student *)b;
return (student1->score > student2->score) ? 1 : ((student1->score == student2->score) ? 0 : -1);
}
// 主函数
int main() {
// 创建三个学生数据
Student students[3] = {
{"张三", 85},
{"李四", 90},
{"王五", 87}
};
// 对学生数组按照成绩降序排序
qsort(students, sizeof(students) / sizeof(students[0]), sizeof(Student), compare);
// 打印排名结果
for (int i = 0; i < 3; i++) {
printf("%d. %s: %.1f分\n", i + 1, students[i].name, students[i].score);
}
return 0;
}
```
在这个例子中,`compare` 函数用于比较学生的分数,`qsort` 函数负责对数组进行排序。注意这里我们假设了成绩越高排名越靠前。
阅读全文
相关推荐
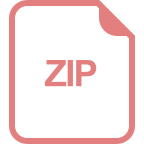
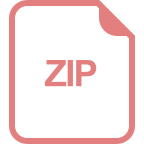
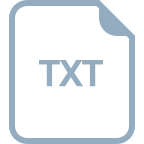
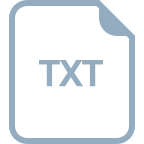
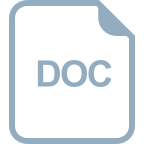
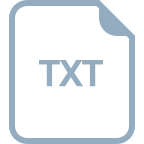
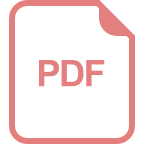
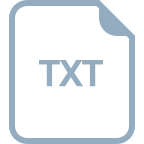
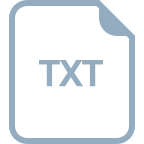
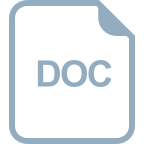
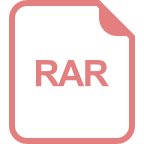
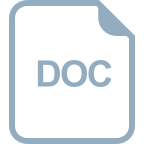
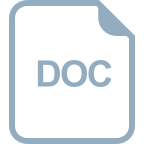

