上述代码在哪个地方读入图片
时间: 2023-12-24 18:05:53 浏览: 110
对不起,我在之前的回答中确实没有提到如何读取图片。如果您不使用OpenCV等库函数,可以使用C或C++的文件读写函数和自己编写的图像解码函数来读取和处理图像数据。
以下是一个基本的读取BMP图像的代码示例:
```c++
#include <fstream>
#include <iostream>
// BMP文件头结构体
#pragma pack(push, 1) // 结构体按字节对齐,不加这个会对齐到4字节
struct BMPHeader {
uint16_t bfType; // 文件类型,必须为0x4d42
uint32_t bfSize; // 文件大小,单位为字节
uint16_t bfReserved1;
uint16_t bfReserved2;
uint32_t bfOffBits; // 数据偏移量,即位图数据的起始位置
};
#pragma pack(pop)
// BMP信息头结构体
struct BMPInfoHeader {
uint32_t biSize; // 信息头大小,一般为40
int32_t biWidth; // 图像宽度,单位为像素
int32_t biHeight; // 图像高度,单位为像素
uint16_t biPlanes; // 颜色平面数,一般为1
uint16_t biBitCount; // 每个像素的位数,一般为24(RGB)
uint32_t biCompression; // 压缩类型,一般为0(不压缩)
uint32_t biSizeImage; // 图像数据大小,单位为字节
int32_t biXPelsPerMeter; // 水平分辨率,单位为像素/米
int32_t biYPelsPerMeter; // 垂直分辨率,单位为像素/米
uint32_t biClrUsed; // 颜色索引数,一般为0
uint32_t biClrImportant; // 重要颜色索引数,一般为0
};
// 读取BMP图像数据
unsigned char* read_bmp(const char* filename, int& width, int& height) {
std::ifstream file(filename, std::ios::binary);
if (!file) {
std::cerr << "Error: cannot open file \"" << filename << "\"" << std::endl;
return nullptr;
}
BMPHeader header;
BMPInfoHeader info_header;
// 读取文件头和信息头
file.read((char*)&header, sizeof(header));
file.read((char*)&info_header, sizeof(info_header));
// 检查文件类型是否为BMP
if (header.bfType != 0x4d42) {
std::cerr << "Error: \"" << filename << "\" is not a BMP file" << std::endl;
return nullptr;
}
// 检查位图压缩类型是否为无压缩
if (info_header.biCompression != 0) {
std::cerr << "Error: \"" << filename << "\" is a compressed BMP file" << std::endl;
return nullptr;
}
// 读取图像数据
width = info_header.biWidth;
height = info_header.biHeight;
int row_size = ((width * info_header.biBitCount + 31) / 32) * 4;
unsigned char* data = new unsigned char[row_size * height];
file.seekg(header.bfOffBits, std::ios::beg);
for (int y = 0; y < height; ++y) {
file.read((char*)(data + row_size * y), row_size);
}
// 翻转图像
int half_height = height / 2;
for (int y = 0; y < half_height; ++y) {
int swap_y = height - y - 1;
for (int x = 0; x < width; ++x) {
int i1 = row_size * y + x * 3;
int i2 = row_size * swap_y + x * 3;
std::swap(data[i1], data[i2]);
std::swap(data[i1 + 1], data[i2 + 1]);
std::swap(data[i1 + 2], data[i2 + 2]);
}
}
return data;
}
int main() {
int width, height;
unsigned char* data = read_bmp("image.bmp", width, height);
if (!data) {
return 1;
}
// 在这里添加计算均值、方差和直方图的代码
delete[] data;
return 0;
}
```
在这个例子中,我们使用了一些文件读写函数和自己编写的BMP解码函数来读取和处理图像数据。这个代码演示了如何读取BMP图像数据,并可以在其中添加计算均值、方差和直方图的代码。您可以根据需要修改代码,以适应其他类型的图像文件。希望这可以帮助您解决问题!
阅读全文
相关推荐
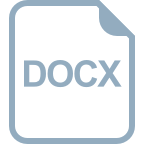
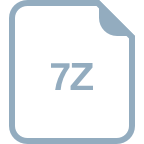
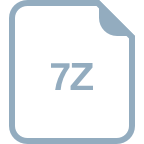
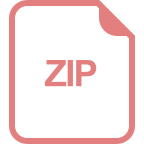
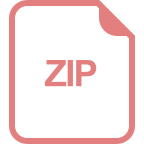
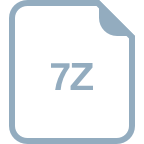
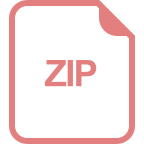
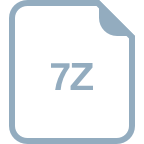
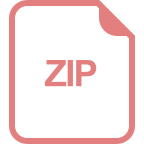
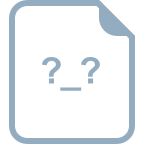