c++定义时间(CMyTime),有小时、分钟和秒三个成员变量,重载 “-” (减)、后置“++”和“>=”运算符,实现函数 show 显示时间,测试 MyTime。
时间: 2023-10-22 09:05:01 浏览: 69
好的,这是一个 C++ 定义时间的例子,包括重载运算符和实现 show 函数:
```c++
#include <iostream>
using namespace std;
class CMyTime {
private:
int hour;
int minute;
int second;
public:
CMyTime(int h, int m, int s) : hour(h), minute(m), second(s) {}
// 重载 "-" 运算符
CMyTime operator-(const CMyTime &t) {
int h = hour - t.hour;
int m = minute - t.minute;
int s = second - t.second;
if (s < 0) {
s += 60;
m--;
}
if (m < 0) {
m += 60;
h--;
}
return CMyTime(h, m, s);
}
// 重载后置 "++" 运算符
CMyTime operator++(int) {
CMyTime t = *this;
second++;
if (second >= 60) {
second -= 60;
minute++;
}
if (minute >= 60) {
minute -= 60;
hour++;
}
return t;
}
// 重载 ">=" 运算符
bool operator>=(const CMyTime &t) {
if (hour > t.hour) {
return true;
} else if (hour < t.hour) {
return false;
} else if (minute > t.minute) {
return true;
} else if (minute < t.minute) {
return false;
} else if (second >= t.second) {
return true;
} else {
return false;
}
}
// 显示时间
void show() {
cout << hour << ":" << minute << ":" << second << endl;
}
};
int main() {
CMyTime t1(10, 20, 30);
CMyTime t2(8, 15, 45);
cout << "t1 = ";
t1.show();
cout << "t2 = ";
t2.show();
cout << "t1 - t2 = ";
(t1 - t2).show();
cout << "t1 >= t2: " << (t1 >= t2) << endl;
cout << "t1++ = ";
(t1++).show();
cout << "t1 = ";
t1.show();
return 0;
}
```
在这个例子中,我们定义了一个 CMyTime 类来表示时间。这个类包含了三个成员变量:小时、分钟和秒。我们重载了减运算符(-),后置递增运算符(++)和大于等于运算符(>=),并实现了一个 show 函数来显示时间。
在 main 函数中,我们创建了两个 CMyTime 对象 t1 和 t2,并显示了它们的值。然后,我们演示了如何使用重载的运算符来计算两个时间之间的差异,并显示了结果。我们还演示了如何使用重载的运算符来比较两个时间的大小,并在控制台上显示结果。
最后,我们演示了如何使用重载的后置递增运算符来增加一个时间的秒数,并在控制台上显示结果。
相关推荐
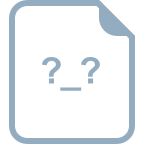
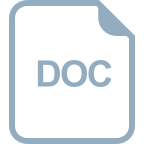














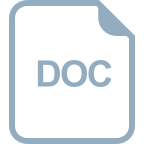